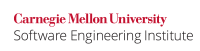
...
Code Block | ||
---|---|---|
| ||
public class AtomicAdder { public synchronized void update(BigInteger f, BigInteger s){ first.set(f); second.set(s); } public synchronized BigInteger add() { return first.get().add(second.get()); } } |
Prefer using the block form of synchronization for better performance, when there are nonatomic operations within the method that do not require any synchronization. These operations can be decoupled from those that require synchronization and executed outside the synchronized
block.
...
Code Block | ||
---|---|---|
| ||
class RaceCollection {
private final List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>());
public void addIPAddress(InetAddress ia) {
// Validate ia
ips.add(ia);
}
public void addAndPrintIP() throws UnknownHostException {
addIPAddress(InetAddress.getLocalHost());
InetAddress[] ia = (InetAddress[]) ips.toArray(new InetAddress[0]);
System.out.println("Number of IPs: " + ia.length);
}
}
|
...