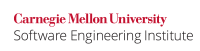
...
Because the check for integer overflow following the addition is absent, the caller must ensure that the increment()
method is called no more than Integer.MAX_VALUE
times for any key. Refer to INT00-J. Perform explicit range checking to ensure integer operations do not overflow for more information.
Compliant Solution (
...
atomic method)
To ensure atomicity, this compliant solution uses a private final lock object to synchronize the statements of the increment()
and getCount()
methodsmethod that guarantees atomicity (AtomicInteger.incrementAndGet()). This provides a happens-before relationship between reading and writing any integer values in the map.
Code Block | ||
---|---|---|
| ||
final class KeyedCounter { private final ConcurrentMap<String, AtomicInteger> map = new ConcurrentHashMap<String, AtomicInteger>(); public void increment(String key) { AtomicInteger value = new AtomicInteger(0); AtomicInteger old = map.putIfAbsent(key, value); if (old != null) { value = old; } value.incrementAndGet(); // Increment the value atomically } public Integer getCount(String key) { AtomicInteger value = map.get(key); return value.get(); } // Other accessors ... } |
...