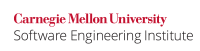
...
Returning references to private
data to untrusted code can be more pernicious than returning references to trusted code. If a class defines a clone()
method that trusted code can use to pass defensive copies of the instance to untrusted code (OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely), the implementing class may violate this guideline. However, the burden is now transferred to the trusted code as it is expected to reliably call the clone()
method before operating on the instance or passing it to untrusted code.
Note that the performance costs of violating this guideline (or calling clone()
) might be significantly more than using an accessor method that returns a copy of a single mutable member. This is because a well designed clone()
method returns a copy of the complete object, including all mutable components) as opposed to that of a single member, which makes it relatively slower.
Wiki Markup |
---|
Pugh \[[Pugh 09|AA. Java References#Pugh 09]\] cites a vulnerability discovered by the Findbugs static analysis tool in the early betas of jdk 1.7. The class {{sun.security.x509.InvalidityDateExtension}} returned a {{Date}} instance through a {{public}} accessor, without creating defensive copies. |
Noncompliant Code Example
...
If the hash table contained references to mutable data such as a series of Date
objects, every one of those objects must be copied by using a copy constructor or method. For further details, refer to FIO31-J. Defensively copy mutable inputs and mutable internal components and OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely. Note that the keys of a hash table need not be deep copied; shallow copying of the references suffices because a hash table's contract dictates that it cannot hold duplicate keys.
Noncompliant Code Example
This noncompliant code example shows a getDate()
accessor method that returns the sole instance of the private Date
object. An untrusted caller will be able to manipulate the instance as it exposes internal mutable components beyond the trust boundaries of the class.
Code Block | ||
---|---|---|
| ||
class MutableClass {
private Date d;
public MutableClass() {
d = new Date();
}
protected Date getDate() {
return d;
}
}
|
Compliant Solution
Do not carry out defensive copying using the clone()
method in constructors, where the (non-system) class can be subclassed by untrusted code. This will limit the malicious code from returning a crafted object when the object's clone()
method is invoked.
Despite this advice, this compliant solution recommends returning a clone of the Date
object. While this should not be done in constructors, it is permissible to use it in accessors. This is because there is no danger of a malicious subclass extending the internal mutable Date
object (Date
is a system class, therefore safe).
Code Block | ||
---|---|---|
| ||
protected Date getDate() { return (Date)d.clone(); } |
Arrays of primitive types are not exempt from this guideline. As arrays are objects in Java, if a reference to an array is returned, the caller may freely modify the contents of the original array. Shallow copies of arrays of primitive types are safe to return.
...