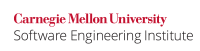
Mutable classes are those which when instantiated, provide return a reference such that the contents of the class instance can be altered by external code. It is important to provide means for creating copies of mutable class members so as classes to allow safe passing in and returning of their respective class objects to untrusted code. The copying ensures that untrusted code cannot manipulate the object's state. The copying mechanism can be offered by overriding java.lang.Object
's clone()
method. However, providing a clone()
method by itself, does not eliminate the need to create defensive copies when receiving input from untrusted code and returning values to the same. Only a trusted caller can be expected to responsibly call the overriding clone()
method before passing instances to untrusted code.
Noncompliant Code Example
In this noncompliant code example, MutableClass
uses declares a mutable Date
object. If the trusted caller passes the Date
instance to untrusted code, and the latter changes the instance of the Date
object (like incrementing the month or changing the timezone), the class implementation no longer remains consistent with its old state. Both, the constructor as well as the getDate
method are susceptible to abuse. This also defies attempts to implement thread safetyThis problem can also arise in the presence of multiple threads.
Code Block | ||
---|---|---|
| ||
public final class MutableClass { private Date d; public MutableClass(Date d) { this.d = d; } public Date getDate() { return d; } } |
...
Compliant Solution
Always provide mechanisms to create copies of the instances of a mutable class. This compliant solution implements the Cloneable
interface and overrides the clone
method to create a deep copy of both the object and the contained mutable Date
object. Since using clone()
independently only produces a shallow copy and still leaves the class mutable. If it is possible that the accessor methods can be called from untrusted code, it is also advisable to also copy all the referenced mutable objects that are passed in before they are stored in the class (FIO31-J. Defensively copy mutable inputs and mutable internal components) or returned from any methods (OBJ37-J. Do not return references to private data). This is because untrusted code cannot be expected to call the object's clone()
method to operate on the copy. This compliant solution not only provides a clone()
method for trusted code but also guarantees that the state of the object cannot be compromised when the accessor method is called directly from untrusted code.
Code Block | ||
---|---|---|
| ||
public final class CloneClass implements Cloneable { private Date d; public CloneClass(Date d) { this.d = new Date(d.getTime()); //copy-in } public Object clone() throws CloneNotSupportedException { final CloneClass cloned = (CloneClass)super.clone(); cloned.d = (Date)d.clone(); //copy mutable Date object manually return cloned; } public Date getDate() { return (Date)d.clone(); //copy and return } } |
Wiki Markup |
---|
At times, a mutable class's member class is declared {{final}} with no accessible copy methods. Callers can use the {{clone()}} method to obtain an instance of the |
Wiki Markup |
---|
Sun's Secure Coding Guidelines document mutable class. This {{clone()}} method must create a new instance of the {{final}} member class and copy the original state to it. The new instance is necessary because no accessible copy method may be available in the member class. If the member class evolves in the future, it is critical to include the new state in the manual copy. \[[SCG 07|AA. Java References#SCG 07]\] provides some very useful tips: |
...
Exceptions
EX1: Sensitive classes should not be made cloneable. (MSC37-J. Make sensitive classes noncloneable)
Risk Assessment
Creating a mutable class without a clone
method may result in the data of the class its instance becoming corrupted by accessing when the instance is passed to untrusted code.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OBJ36- J | low | likely | low | P9 | L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] [method clone()|http://java.sun.com/javase/6/docs/api/java/lang/Object.html#clone()] \[[Security 06|AA. Java References#Security 06]\] \[[SCG 07|AA. Java References#SCG 07]\] Guideline 2-2 Support copy functionality for a mutable class \[[Bloch 08|AA. Java References#Bloch 08]\] Item 39: Make defensive copies when needed \[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 374|http://cwe.mitre.org/data/definitions/374.html] "Mutable Objects Passed by Reference", [CWE ID 375|http://cwe.mitre.org/data/definitions/375.html] "Passing Mutable Objects to an Untrusted Method" |
...