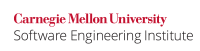
Mutable classes are those which when instantiated, provide a reference such that the contents of the class can be altered anytimeby external code. It is important to provide means for creating copies of mutable class instances members so as to allow safe passing in and returning of their respective class objects when used within method arguments.
Noncompliant Code Example
In this noncompliant code example, MutableClass
uses a mutable Date
object. If the caller changes the instance of the Date
object (like incrementing the month), the class implementation no longer remains consistent with its old state. Both, the constructor as well as the getDate
method are susceptible to abuse. This also defies attempts to implement thread safety.
...
This code also violates OBJ37-J. Do not return references to private data.
Compliant Solution
Always provide mechanisms to create copies of the instances of a mutable class. This compliant solution implements the Cloneable
interface and overrides the clone
method to create a deep copy of both the object and the contained mutable Date
object. Since using clone()
independently only produces a shallow copy and still leaves the class mutable, it is advised advisable to also copy all the referenced mutable objects that are passed in or returned from any method.
Code Block | ||
---|---|---|
| ||
public final class CloneClass implements Cloneable { private Date d; public CloneClass(Date d) { this.d = new Date(d.getTime()); //copy-in } public Object clone() throws CloneNotSupportedException { final CloneClass cloned = (CloneClass)super.clone(); cloned.d = (Date)d.clone(); //copy mutable Date object manually return cloned; } public Date getDate() { return (Date)d.clone(); //copy and return } } |
At times, a class is labeled declared final
with no accessible copy methods. Callers can then use the clone()
method to obtain an instance of the class, create a new instance with the original state and subsequently proceed to use it. Similarly, mutable objects obtained must also be copied when necessary.
Wiki Markup |
---|
Sun's Secure Coding Guidelines document \[[SCG 07|AA. Java References#SCG 07]\] provides some very useful tips: |
If a class is
final
and does not provide an accessible method for acquiring a copy of it, callers can resort to performing a manual copy. This involves retrieving state from an instance of that class, and then creating a new instance with the retrieved state. Mutable state retrieved during this process must likewise be copied if necessary. Performing such a manual copy can be fragile. If the class evolves to include additional state in the future, then manual copies may not include that state.
...
Creating a mutable class without a clone
method may result in the data of the class becoming corrupted by accessing code.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OBJ36- J | low | likely | low | P9 | L2 |
...