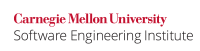
The synchronized
keyword is used to acquire a mutual-exclusion lock so that no other thread can acquire the lock, while it is being held by the executing thread. Recall that there are two ways to synchronize access to shared mutable variables, method synchronization and block synchronization. Excessive method synchronization can induce a denial of service (DoS) vulnerability because another class whose member locks on the class object, can fail to release the lock promptly. However, this requires the victim class to be accessible to the hostile class.
Wiki Markup |
---|
The _private lock object_ idiom can be used to prevent the DoS vulnerability. The keyword idiom consists of a {{private}} object declared as an instance field. The {{private}} object must be explicitly used for locking purposes in {{synchronized}} is known to make sure the synchronization of threads within a process in Java. However, care should be taken when writing a thread-safe class to defend itself from possible denial-of-service attack \[[Effective Java, Item 52|http://books.google.com/books?id=ZZOiqZQIbRMC&pg=PA210&lpg=PA210&dq=private+lock+object+idiom&source=bl&ots=UZP26thN4-&sig=QCPUutihieMlD8cNJIwPLpvBCXc&hl=en&ei=RJPzSsG0EJXilAezjuWjAw&sa=X&oi=book_result&ct=result&resnum=1&ved=0CAgQ6AEwAA#v=onepage&q=private%20lock%20object%20idiom&f=false]\]. |
A idiom called private lock object can be used to prevent the DOS attack. By calling the synchronized
block, the caller is only able to obtain a lock object in the instance, not the whole instance itself so that other threads may still proceed. This technique also enhance concurrency granularity as different locks can be created according to the program logic and resources.
Internal object for locking is especially suitable in a inheritance environment. If the superclass use the entire instance for locking, a subclass can interfere with its operation. Declare lock as member variable eliminate this situation.
Noncompliant Code Example
blocks, within the class's methods. This lock object belongs to an instance of the object and is not associated with the object itself. Consequently, there is no lock contention between a class method and a method of the hostile class when both try to lock on the class object. \[[Bloch 01|AA. Java References#Bloch 01]\] |
This idiom can also be suitably used by classes designed for inheritance. If a superclass thread requests a lock on the class object's monitor, a subclass thread can interfere with its operation. Refer to the guideline CON36-J. Always synchronize on the appropriate object for more details.
Noncompliant Code Example
This noncompliant code example exposes the class object someObject
to untrusted code. The untrusted code attempts to acquire a lock on the class object's monitor and upon succeeding, introduces an indefinite delay which holds up the synchronized
changeValue()
method from acquiring the same lock. Note that the untrusted code also violates CON07-J. Do not defer a thread that is holding a lock A malicious client using the instantiated thread-safe object can mount a denial-of-service attack simply by holding the lock on the object. Notice that this also conflict with \[[CON07-J. Do not defer a thread that is holding a lock|CON07-J. Do not defer a thread that is holding a lock]\]. Wiki Markup
Code Block | ||
---|---|---|
| ||
public class importantObjSomeObj { public synchronized void changeValue() { // lockLocks on this the class object's monitor // ... } ...} } // Denial-of-service attack from caller synchronized (importantObjectUntrusted code synchronized (someObject) { while(true) { Thread.sleep(Integer.MAX_VALUE); // Indefinitely delay someObject Disable importantObject} } |
Compliant Solution
Thread-safe classes that use intrinsic synchronization may be protected by using the private lock object idiom and adapting them to use block synchronization. In this compliant solution, if the method changeValue()
is called, the lock is obtained on a private
Object
that is both invisible and inaccessible from the caller.
Code Block | ||
---|---|---|
| ||
// private lock object idiom - thawts denial-of-service attack public class importantObjSomeObj { private final Object lock = new Object(); // private lock object public void changeValue() { // lock on this synchronized(lock) { // Locks on the private Object // ... } } ... } |
If the method changeValue
is called in this case, the lock is obtained on a private Object
that is both invisible and inaccessible from the caller. The this
instance is not vulnerable from denial-of-service attack. Thread-safe class may be protected in this way by using the private lock object idiom.
The details of using private object lock can refer to \[[CON36-J. Always synchronize on the appropriate object|For more details on using the Wiki Markup private
Object lock refer to CON36-J. Always synchronize on the appropriate object]\].
Risk Assessment
Synchronizing on the whole instance Exposing the class object to untrusted code can result in Denialdenial-of-service and private object lock is preferred when possible.
Rule Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON10- J | low | probable | medium | P4 | L3 |
...
References
Wiki Markup |
---|
\[[EffectiveBloch 01|AA. Java, References#Bloch 01]\] Item 52|http://books.google.com/books?id=ZZOiqZQIbRMC&pg=PA210&lpg=PA210&dq=private+lock+object+idiom&source=bl&ots=UZP26thN4-&sig=QCPUutihieMlD8cNJIwPLpvBCXc&hl=en&ei=RJPzSsG0EJXilAezjuWjAw&sa=X&oi=book_result&ct=result&resnum=1&ved=0CAgQ6AEwAA#v=onepage&q=private%20lock%20object%20idiom&f=false]\]: "Document Thread Safety" |
...
CON08-J. Ensure that threads do not fail during activation 11. Concurrency (CON) CON30-J. Synchronize access to shared mutable variables