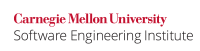
...
Code Block | ||
---|---|---|
| ||
class FinalClass{ private int a; private int b; FinalClass(int a, int b){ this.a = a; this.b = b; } void set_ab(int a, int b){ this.a = a; this.b = b; } void print_ab(){ System.out.println("the value a is: "+ this.a); System.out.println("the value b is: "+ this.b); } } public class FinalCaller { public static void main(String[] args) { final FinalClass fc = new FinalClass(1,2); fc.print_ab(); //now we change the value of a,b. fc.set_ab(5, 6); fc.print_ab(); } } |
...
Compliant Solution
If a
and b
have to be kept immutable after their initialization, the simplest approach is to declare them as final
.
Code Block | ||
---|---|---|
| ||
private final int a; private final int b; void set_ab(int a, int b){ //But now the compiler complains about set_ab method this.a = a; this.b = b; } |
Unfortunately, now one cannot have setter methods of The problem with this method is that setter methods cannot be used to alter a
and b
.
Compliant Solution
An alternative approach is to provide a clone
method in the class. The clone method can be used to get a copy of the original object. This new object can be freely used without affecting the original object.
...
The class is made final to prevent subclasses from overriding the clone()
method. This enables the class to be accessed and used, while preventing the fields from being modified, and complies with OBJ36-J. Provide mutable classes with a clone method.
Noncompliant Code Example
Another common mistake is to use a public static final
array. Clients can trivially modify the contents of the array (although they will not be able to change the array itself, as it is final).
...
Code Block | ||
---|---|---|
| ||
public static final SomeType [] SOMETHINGS = { ... }; |
Compliant Solution
One approach is to make use of the above method: first define a private
array and then provide a public
method that returns a copy of the array.
...
Now the original array values cannot be modified by a client.
Compliant Solution
An alternative approach is to have a private
array from which a public
immutable list is constructed:
...