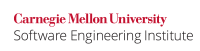
...
Code Block | ||
---|---|---|
| ||
class Inventory { private final Hashtable<StringHashtable<String, Integer>Integer> items; public Inventory() { items = new Hashtable<StringHashtable<String, Integer>Integer>(); } public List<String>List<String> getStock() { List<String>List<String> l = new ArrayList<String>ArrayList<String>(); Enumeration e = items.keys(); while(e.hasMoreElements()) { Object value = e.nextElement(); if((items.get(value)) == 0) { l.add((String)value); } } if(items.size() == 0) { return null; } else { return l; } } } public class Client { public static void main(String[] args) { Inventory iv = new Inventory(); List<String>List<String> items = iv.getStock(); System.out.println(items.size()); // Throws a NPE } } |
...
Code Block | ||
---|---|---|
| ||
class Inventory { private final Hashtable<StringHashtable<String, Integer>Integer> items; public Inventory() { items = new Hashtable<StringHashtable<String, Integer>Integer>(); } public List<String>List<String> getStock() { List<String>List<String> l = new ArrayList<String>ArrayList<String>(); Integer noOfItems; // Number of items left in the inventory Enumeration e = items.keys(); while(e.hasMoreElements()) { Object value = e.nextElement(); if((noOfItems = items.get(value)) == 0) { l.add((String)value); } } return l; // Return list (possibly zero-length) } } public class Client { public static void main(String[] args) { Inventory iv = new Inventory(); List<String>List<String> items = iv.getStock(); System.out.println(items.size()); // Does not throw a NPE } } |
...
Code Block | ||
---|---|---|
| ||
public List<String>List<String> getStock() { List<String>List<String> l = new ArrayList<String>ArrayList<String>(); Integer noOfItems; // Number of items left in the inventory Enumeration e = items.keys(); while(e.hasMoreElements()) { Object value = e.nextElement(); if((noOfItems = items.get(value)) == 0) { l.add((String)value); } } if(l.isEmpty()) { return Collections.EMPTY_LIST; // Always zero-length } else { return l; // Return list } } // Class Client ... |
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[Bloch 08|AA. Java References#Bloch 08]\] Item 43: return empty arrays or collections, not nulls |
...
MET02-J. Avoid ambiguous uses of overloading 12. Methods (MET) MET04-J. Always provide feedback about the resulting value of a method