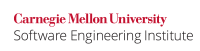
...
Code Block | ||
---|---|---|
| ||
class Inventory { private final Hashtable<String, Integer> items; public Inventory() { items = new Hashtable<String, Integer>(); } public ArrayList<String>List<String> getStock() { ArrayList<String>List<String> l = new ArrayList<String>(); Enumeration e = items.keys(); while(e.hasMoreElements()) { Object value = e.nextElement(); if((items.get(value)) == 0) { l.add((String)value); } } if(items.size() == 0) { return null; } else { return l; } } } public class Client { public static void main(String[] args) { Inventory iv = new Inventory(); ArrayList<String>List<String> items = iv.getStock(); System.out.println(items.size()); // throwsThrows a NPE } } |
Compliant Solution
...
Code Block | ||
---|---|---|
| ||
class Inventory { private final Hashtable<String, Integer> items; public Inventory() { items = new Hashtable<String, Integer>(); } public ArrayList<String>List<String> getStock() { ArrayList<String>List<String> l = new ArrayList<String>(); Integer noOfItems; // Number of items left in the inventory Enumeration e = items.keys(); while(e.hasMoreElements()) { Object value = e.nextElement(); if((noOfItems = items.get(value)) == 0) { l.add((String)value); } } return l; // Return list (possibly zero-length) } } public class Client { public static void main(String[] args) { Inventory iv = new Inventory(); ArrayList<String>List<String> items = iv.getStock(); System.out.println(items.size()); // Does not throw a NPE } } |
...
Code Block | ||
---|---|---|
| ||
public ArrayList<String>List<String> getStock() { ArrayList<String>List<String> l = new ArrayList<String>(); Integer noOfItems; // Number of items left in the inventory Enumeration e = items.keys(); while(e.hasMoreElements()) { Object value = e.nextElement(); if((noOfItems = items.get(value)) == 0) { l.add((String)value); } } if(l.isEmpty()) { return Collections.EMPTY_LIST; // Always zero-length } else { return l; // Return list } } // Class Client ... |
...