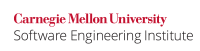
...
This noncompliant code example attempts to guard access to the static counter
field using a non-static lock object. When two Runnable
tasks are started, they create two instances of the lock object and lock on each instance separately.
Code Block | ||
---|---|---|
| ||
public final class CountBoxes implements Runnable {
private static volatile int counter;
// ...
private final Object lock = new Object();
@Override public void run() {
synchronized (lock) {
counter++;
// ...
}
}
public static void main(String[] args) {
for (int i = 0; i < 2; i++) {
new Thread(new CountBoxes()).start();
}
}
}
|
...
This noncompliant code example uses method synchronization to protect access to a static class counter
field.
Code Block | ||
---|---|---|
| ||
public final class CountBoxes implements Runnable {
private static volatile int counter;
// ...
public synchronized void run() {
counter++;
// ...
}
// ...
}
|
...
This compliant solution ensures the atomicity of the increment operation by locking on a static object.
Code Block | ||
---|---|---|
| ||
public class CountBoxes implements Runnable {
private static int counter;
// ...
private static final Object lock = new Object();
public void run() {
synchronized (lock) {
counter++;
// ...
}
}
// ...
}
|
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
LCK06-J | medium | probable | medium | P8 P0 | L2 |
Automated Detection
Some static analysis tools can detect violations of this rule.
...
Tasklist | ||||
---|---|---|---|---|
| ||||
||Completed||Priority||Locked||CreatedDate||CompletedDate||Assignee||Name|| |T|M|F|1270215165305|1271447005294|rcs_mgr|"Ideally, the lock should also be private and final" => I have been penalized for using "ideally" before. I would just remove that sentence or make it more definitive. Note that we also have an NCE that uses method synchronization. Perhaps we also need a CS with a static method.| |