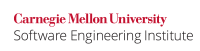
...
Because the HttpServlet
class is a singleton, there is only one last
field that is shared by every client who accesses the servlet. Therefore the contents of the last
field can be the previous setting of the field by a different client. (Because Also, since there is no thread-safety, it is possible for the last
field to take on a stale value should two clients request the parameter simultaneously.)
Noncompliant Code Example
...
Code Block | ||||
---|---|---|---|---|
| ||||
public class SampleServlet extends HttpServlet {
private static String last = "last";
// ... other methods unchanged
}
|
Compliant Solution
In this compliant solution, the last
field is static, and is protected from concurrent access by a separate lock object, as is recommended by LCK00-J. Use private final lock objects to synchronize classes that may interact with untrusted code. This guarantees thread-safety in the servlet. The servlet can still return the last parameter entered by a different session, however.
Code Block | ||||
---|---|---|---|---|
| ||||
public class SampleServlet extends HttpServlet { private static String last = "last"; private static final Object lastLock = new Object(); public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException { response.setContentType("text/html"); PrintWriter out = response.getWriter(); out.println("<html>"); String current = request.getParameter("current"); if (current != null) { out.println("Current Parameter:"); out.println(sanitize(current)); synchronized (lock) { out.println("<br>Last Parameter:"); out.println(sanitize(last)); } }; out.println("<p>"); out.print("<form action=\""); out.print("SampleServlet\" "); out.println("method=POST>"); out.println("Parameter:"); out.println("<input type=text size=20 name=current>"); out.println("<br>"); out.println("<input type=submit>"); out.println("</form>"); synchronized (lock) { last = current; } } public void doPost(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException { doGet(request, response); } // Filter the specified message string for characters // that are sensitive in HTML. public static String sanitize(String message) { // ... } } |
Compliant Solution
This compliant solution stores the last parameter in the HttpSession
object, which is provided as part of the HttpServletRequest
. The servlet mechanism keeps track of the session, providing the client with the session's ID, which is stored as a cookie by the client's browser. The other information in the session, including the last
attribute, are stored by the server. Consequently, the servlet provides the last value that was presented to the servlet in the same session (avoiding race conditions with requests from other sessions). The local variables, which temporarily hold data in this example, are not vulnerable to race conditions in the singleton.
...