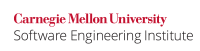
Wiki Markup |
---|
According to Goetz and colleagues \[[Goetz 2006|AA. Bibliography#Goetz 06]\]: |
Client-side locking entails guarding client code that uses some object X with the lock X uses to guard its own state. In order to use client-side locking, you must know what lock X uses.
...
Wiki Markup |
---|
The documentation of a class that supports client-side locking should explicitly state its applicability. For example, the class {{java.util.concurrent.ConcurrentHashMap<K,V>}} should not be used for client-side locking, because its documentation states that \[[API 2006|AA. Bibliography#API 06]\]: |
... even though all operations are thread-safe, retrieval operations do not entail locking, and there is not any support for locking the entire table in a way that prevents all access. This class is fully interoperable with
Hashtable
in programs that rely on its thread safety but not on its synchronization details.
...
This noncompliant code example uses a thread-safe Book
class that cannot be refactored. Refactoring might be impossible, for example, if the source code is not available for review or if the class is part of a general library that cannot be extended.
Code Block |
---|
final class Book { // MayCould change its locking policy in the future to use private final locks private final String title; private Calendar dateIssued; private Calendar dateDue; Book(String title) { this.title = title; } public synchronized void issue(int days) { dateIssued = Calendar.getInstance(); dateDue = Calendar.getInstance(); dateDue.add(dateIssued.DATE, days); } public synchronized Calendar getDueDate() { return dateDue; } } |
...
Extension is more fragile than adding code directly to a class, because the implementation of the synchronization policy is now distributed over multiple, separately maintained source files. If the underlying class were to change its synchronization policy by choosing a different lock to guard its state variables, the subclass would subtly and silently break , because it no longer used the right lock to control concurrent access to the base class's state.
...
Code Block | ||
---|---|---|
| ||
// This class maycould change its locking policy in the future, for example, // if new non-atomic methods are added class IPAddressList { private final List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public List<InetAddress> getList() { return ips; // No defensive copies required as package-private visibility } public void addIPAddress(InetAddress address) { ips.add(address); } } class PrintableIPAddressList extends IPAddressList { public void addAndPrintIPAddresses(InetAddress address) { synchronized(getList()) { addIPAddress(address); InetAddress[] ia = (InetAddress[]) getList().toArray(new InetAddress[0]); // ... } } } |
...
Wiki Markup |
---|
In this case, composition allows the {{PrintableIPAddressList}} class to use its own intrinsic lock independent of the underlying list class's lock. The underlying collection does not need to be thread-safe because the {{PrintableIPAddressList}} wrapper prevents direct access to its methods by publishing its own synchronized equivalents. This approach provides consistent locking even if the underlying class changes its locking policy in the future \[[Goetz 2006|AA. Bibliography#Goetz 06]\]. |
...