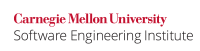
...
Mutable classes must provide either a copy constructor or a public static factory method that returns a copy of an instance. Alternatively, final classes may advertise their copy functionality by overriding java.lang.Object
's clone()
method. Use of the clone()
method is secure only for final
classes; non-final classes are forbidden to use this approach. must not take this approach (see OBJ03-J. Sensitive classes must not let themselves be copied.)
Trusted callers can be trusted to use the provided copy functionality to make defensive copies before passing object instances to untrusted code. Untrusted callers cannot be trusted to make such defensive copies. Consequently, providing copy functionality is insufficient to does not obviate the need for making defensive copies either of inputs received from, or of outputs that are returned to, untrusted code.
Noncompliant Code Example
In this noncompliant code example, MutableClass
uses a mutable field date
of type Date
. Class Date
is also a mutable class. The example is noncompliant because the MutableClass
objects lack copy functionality.
...
When a trusted caller passes an instance of MutableClass
to untrusted code, and the untrusted code modifies that instance (perhaps by incrementing the month or changing the timezone), the state of the object may may consequently become can be made inconsistent with respect to its previous state. Similar problems can arise in the presence of multiple threads, even in the absence of untrusted code.
...
When untrusted code can call accessor methods and can pass passing mutable arguments, make create defensive copies of the arguments before they are stored in any instance fields. See guideline FIO00-J. Defensively copy mutable inputs and mutable internal components for additional information. When retrieving internal mutable state, make a defensive copy of that state before returning it to untrusted code. See guideline OBJ09-J. Defensively copy private mutable class members before returning their references for additional information.
These defensive Defensive copies would be are unnecessary if untrusted code always invoked invokes object's clone()
method on mutable state received from mutable classes and then operated only on the cloned copy. Unfortunately, untrusted code has little incentive to do so, and malicious code has every incentive to misbehave. This compliant solution both provides a clone()
method to trusted code and also guarantees that the state of the object cannot be compromised when the accessor methods are called directly from untrusted code.
...
Code Block | ||
---|---|---|
| ||
public final class MutableClass implements Cloneable { private final Date date; // final field public MutableClass(Date d) { this.date = new Date(d.getTime()); // copy-in } public Date getDate() { return (Date)date.clone(); // copy and return } public Object clone() throws CloneNotSupportedException { Date d = (Date)date.clone(); MutableClass cloned = new MutableClass(d); return cloned; } } |
...
Mutable classes that define a clone()
method must be declared final
.
Exceptions
OBJ08-EX0: Sensitive classes should not be cloneable, as per guideline OBJ03-J. Sensitive classes must not let themselves be copied.
...