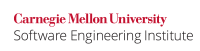
...
This means that a method may use uninitialized data and cause that causes runtime exceptions or lead leads to unanticipated outcomes. Calling overridable methods from constructors can also result in the escaping of the this
reference before construction has concluded. (See guideline TSM01-J. Do not let the (this) reference escape during object construction.)
Noncompliant Code Example
In addition to constructors, do not call overridable methods from the clone()
, readObject()
and readObjectNoData()
methods as it would allow attackers to obtain partially initialized instances of classes. (See guidelines MET07-J. Do not invoke overridable methods on the clone under construction and SER11-J. Do not invoke overridable methods from the readObject method.) It is also insecure to call an overridden method from the finalize()
method. This can prolong the subclass' life and in fact, render the finalization call useless (see the example in guideline MET18-J. Avoid using finalizers.) Additionally, if the subclass's finalizer has terminated key resources, invoking its methods from the superclass may lead one to observe the object in an inconsistent state and in the worst case result in a NullPointerException
.
Noncompliant Code Example
This noncompliant code example results in the use of uninitialized data by the doLogic()
methodThis noncompliant code example invokes the doLogic()
method from the class constructor. The superclass's doLogic()
method gets invoked on the first call, however, the overriding method is invoked on the second one. In the second call, the issue is that the constructor for SubClass
initiates the super class's constructor which undesirably ends up calling SubClass
's doLogic()
method. The value of color
is left as null
because the initialization of the class SubClass
has not yet concluded.
Code Block | ||
---|---|---|
| ||
class BaseClassSuperClass { public BaseClassSuperClass () { doLogic(); } public void doLogic() { System.out.println("This is super-class!"); } } class SubClass extends BaseClassSuperClass { private String color = null; public SubClass() { super(); color = "Red"; } public void doLogic() { // Color becomes null System.out.println("This is sub-class! The color is :" + color); // ... } } public class Overridable { public static void main(String[] args) { BaseClassSuperClass bc = new BaseClassSuperClass(); // Prints "This is super-class!" BaseClassSuperClass sc = new SubClass(); // Prints "This is sub-class! The color is :null" } } |
The doLogic()
method is invoked from the superclass's constructor. When the superclass is constructed directly, the doLogic()
method in the superclass is invoked without issue. However, when the subclass initiates the super class's construction, the subclasses' doLogic()
method is invoked instead. In this case, the value of color
is still null
because the subclasses constructor has not yet concluded.
Compliant Solution
This compliant solution declares the doLogic()
method as final
so that it is no longer overridable.
Code Block | ||
---|---|---|
| ||
class BaseClassSuperClass { public BaseClassSuperClass() { doLogic(); } public final void doLogic() { System.out.println("This is super-class!"); } } |
...
Risk Assessment
Allowing a constructor to call overridable methods may give an attacker access to the this
reference before an object is fully initialized which, in turn, could lead to a vulnerability.
...
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
Wiki Markup |
---|
\[[JLS 2005|AA. Bibliography#JLS 05]\] [Chapter 8, Classes|http://java.sun.com/docs/books/jls/third_edition/html/classes.html], 12.5 "Creation of New Class Instances" \[[SCG 2007|AA. Bibliography#SCG 07]\] Guideline 4-3 Prevent constructors from calling methods that can be overridden \[[ESA 2005|AA. Bibliography#ESA 05]\] Rule 62: Do not call non-final methods from within a constructor. \[[Rogue 2000|AA. Bibliography#Rogue 2000]\] Rule 81: Do not call non-final methods from within a constructor. |
...