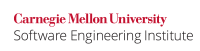
Never use the clone method for defensive copying of untrusted method parameters. Making defensive copies of mutable parameters mitigates against a variety of security vulnerabilities; see OBJ06-J. Defensively copy mutable inputs and mutable internal components for additional information. However, inappropriate use of the clone method can allow an attacker to exploit vulnerabilities by providing arguments that pass initial validation but subsequently return unexpected values. Such objects may consequently bypass validation and security checks. Never use the clone method to make defensive copies of objects that are instances of classes that both are nonfinal and provide a clone()
method.
Noncompliant Code Example
This noncompliant code example defines a validateValue()
method that validates a time value.
Code Block | ||
---|---|---|
| ||
private Boolean validateValue(long time) { // Perform validation return true; // If the time is valid } private void storeDateinDB(java.util.Date date) throws SQLException { final java.util.Date copy = (java.util.Date)date.clone(); if (validateValue(copy.getTime())) { Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... } } |
The storeDateinDB()
method accepts an untrusted date
argument and attempts to make a defensive copy using the clone()
method. The attacker can override the getTime()
method so that it passes validation when called for the first time but provides an unexpected value when it is used a second time.
Code Block |
---|
public class MaliciousDate extends java.util.Date { private static int count = 0; @Override public long getTime() { java.util.Date d = new java.util.Date(); return (count++ == 1) ? d.getTime() : d.getTime() - 1000; } } |
Compliant Solution
This compliant solution avoids using the clone method. Instead, it creates a new java.util.Date
object that is subsequently used for access control checks and for insertion into the database.
Code Block | ||
---|---|---|
| ||
private void storeDateinDB(java.util.Date date) throws SQLException { final java.util.Date copy = new java.util.Date(date.getTime()); if (validateValue(copy.getTime())) { Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... } } |
Risk Assessment
Using the clone()
method to copy untrusted arguments affords attackers the opportunity to bypass validation and security checks.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET52-JG | high | likely | low | P27 | L1 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
MET07-J. Do not invoke overridable methods on the clone under construction 05. Methods (MET) MET53-J. Always provide feedback about the resulting value of a method