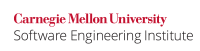
...
This noncompliant code example defines a utility method that accepts a time
argument.
Code Block | ||
---|---|---|
| ||
public synchronized void doSomething(long time)
throws InterruptedException {
// ...
Thread.sleep(time);
}
|
...
This compliant solution defines the doSomething()
method with a timeout
parameter rather than the time
value. Using Object.wait()
instead of Thread.sleep()
allows setting a time out period during which a notification may awaken the thread.
Code Block | ||
---|---|---|
| ||
public synchronized void doSomething(long timeout)
throws InterruptedException {
// ...
while (<condition does not hold>) {
wait(timeout); // Immediately releases the current monitor
}
}
|
...
This noncompliant code example defines a sendPage()
method that sends a Page
object from a server to a client. The method is synchronized to protect the pageBuff
array when multiple threads request concurrent access.
Code Block | ||
---|---|---|
| ||
// Class Page is defined separately.
// It stores and returns the Page name via getName()
Page[] pageBuff = new Page[MAX_PAGE_SIZE];
public synchronized boolean sendPage(Socket socket, String pageName)
throws IOException {
// Get the output stream to write the Page to
ObjectOutputStream out
= new ObjectOutputStream(socket.getOutputStream());
// Find the Page requested by the client
// (this operation requires synchronization)
Page targetPage = null;
for (Page p : pageBuff) {
if (p.getName().compareTo(pageName) == 0) {
targetPage = p;
}
}
// Requested Page does not exist
if (targetPage == null) {
return false;
}
// Send the Page to the client
// (does not require any synchronization)
out.writeObject(targetPage);
out.flush();
out.close();
return true;
}
|
...
In this compliant solution, the unsynchronized sendPage()
method calls the synchronized getPage()
method to retrieve the requested Page
in the pageBuff
array. After the Page
is retrieved, sendPage()
calls the unsynchronized deliverPage()
method to deliver the Page
to the client.
Code Block | ||
---|---|---|
| ||
// No synchronization
public boolean sendPage(Socket socket, String pageName) {
Page targetPage = getPage(pageName);
if (targetPage == null){
return false;
}
return deliverPage(socket, targetPage);
}
// Requires synchronization
private synchronized Page getPage(String pageName) {
Page targetPage = null;
for (Page p : pageBuff) {
if (p.getName().equals(pageName)) {
targetPage = p;
}
}
return targetPage;
}
// Return false if an error occurs, true if successful
public boolean deliverPage(Socket socket, Page page) {
ObjectOutputStream out = null;
boolean result = true;
try {
// Get the output stream to write the Page to
out = new ObjectOutputStream(socket.getOutputStream());
// Send the page to the client
out.writeObject(page);out.flush();
} catch (IOException io) {
result = false;
} finally {
if (out != null) {
try {
out.close();
} catch (IOException e) {
result = false;
}
}
}
return result;
}
|
...
LCK09-EX1: Methods that require multiple locks may hold several locks while waiting for the remaining locks to become available. This constitutes a valid exception, although the programmer must follow other applicable rules, especially rule LCK07-J. Avoid deadlock by requesting and releasing locks in the same order to avoid deadlock.
Automated Detection
Some static analysis tools are capable of detecting violations of this rule.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
ThreadSafe |
| CCE_LK_LOCKED_BLOCKING_CALLS | Implemented |
Risk Assessment
Blocking or lengthy operations performed within synchronized regions could result in a deadlocked or unresponsive system.
...
08. Locking (LCK) LCK10-J. Do not use incorrect forms of the double-checked locking idiom