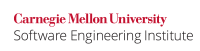
...
Wiki Markup |
---|
The general contract for the {{write()}} method says that it writes one byte to the output stream. The byte to be written constitutes the eight lower order bits of the argument {{b}}, passed to the {{write()}} method. The 24 high-order bits of {{b}} are ignored. \[[API 06|AA. Java References#API 06]\] |
Noncompliant Code Example
This noncompliant code example accepts a value from the user without validating it. Any value that is not in the range of 0 to 255 is truncated. For instance, write(303)
prints /
because the lower order bits of 303 are preserved while the top 24 order bits are lost (303 mod 256 is 47 and /
has ASCII code 47). That is, the result is remainder modulo 256 of the absolute value of the input.
Code Block | ||
---|---|---|
| ||
class ConsoleWrite { public static void main(String[] args) { //Any input value > 255 will result in unexpected output System.out.write(Integer.valueOf(args[0].toString())); System.out.flush(); } } |
Compliant Solution
Use alternative means to output integers such as the System.out.print*
methods.
Code Block | ||
---|---|---|
| ||
class ConsoleWrite { public static void main(String[] args) { System.out.println(args[0]); } } |
Compliant Solution (2)
Alternatively, perform range checking to be compliant. While this particular compliant solution still does not display the original out-of-range integer correctly, it behaves well when the corresponding read()
method is used to convert the byte
value back to a value of type int
. This is because it guarantees that the byte
variable will contain representable data.
...
Note that a NumberFormatException
results when attempting to use the Integer.valueOf()
method, if args0
contains a value that is larger than the maximum value of an int
.
Compliant Solution (3)
This compliant solution uses the writeInt()
method of the DataOutputStream
class.
Code Block | ||
---|---|---|
| ||
class FileWrite { public static void main(String[] args) throws NumberFormatException, IOException { FileOutputStream out = new FileOutputStream("output"); DataOutputStream dos = new DataOutputStream(out); dos.writeInt(Integer.valueOf(args[0].toString())); // close out and dos } } |
Risk Assessment
Using the write()
method to output integers may result in unexpected values.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
INT31- J | low | unlikely | medium | P2 | L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] method [write()|http://java.sun.com/javase/6/docs/api/java/io/OutputStream.html#write(int)] \[[Harold 99|AA. Java References#Harold 99]\] |
...