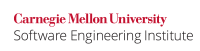
...
An alternative approach is to provide the clone method in the class. When you want do something about the object, you can use clone method to get a copy of original object. Now, you can do everything to this new object, and the original one will be never changed.
Code Block |
---|
 public Test2class NewFinal implements Cloneable {  NewFinal(int a, int b){   this.a = a;   this.b = b;  }  void print_ab(){   System.out.println("the value a is: "+this.a);   System.out.println("the value b is: "+this.b);  }  void set_ab(int a, int b){   this.a = a;   this.b = b;  }  public NewFinal clone() throws CloneNotSupportedException{   Test2  NewFinal cloned = (Test2NewFinal) super.clone();   return cloned;  }  private int a;  private int b; } public class Test2 {   public static void main(String[] args) {        final NewFinal mytest = new NewFinal(1,2);        mytest.print_ab();        //get the copy of original object        try {           NewFinal mytest2 = mytest.clone();        //now we change the value of a,b of the copy.   mytest2.set_ab(5, 6);   //but the original value will not be changed        mytest.print_ab();   } catch (CloneNotSupportedException e) {    // TODO Auto-generated catch block    e.printStackTrace();   }           } } |
Risk Assessment
Using final to declare the reference to an object is a potential security risk, because the contents of the object can still be changed.
...