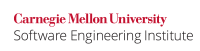
In many times, you may want to create a dynamic array of integers. Unfortunately, the type parameter inside the angle brackets cannot be a primitive type. It is not possible to form an ArrayList<int>. Thanks to the wrapper class, now you can use ArrayList<Integer> to achieve this goal. And the process from int to Integer is called autoboxing. However, you should always be careful about doing this. Take the code below as an example:
Code Example
Code Block |
---|
public class TestWrapper2 { Â public static void main(String[] args) { Â Â Â Â Integer i1 = 100; Â Â Â Â Integer i2 = 100; Â Â Â Â Integer i3 = 1000; Â Â Â Â Integer i4 = 1000; Â Â Â Â System.out.println(i1==i2); Â Â Â Â System.out.println(i3==i4); Â Â Â Â Â } } |
Output of this code
Code Block |
---|
true false |
It is because that in JDK 5.0, if the value p being boxed is true, false, a byte, an ASCII character, or an integer or short number between -127 and 128, then let r1 and r2 be the results of any two boxing conversions of p. It is always the case that r1 == r2. And the reason for this rule explained in criterion for autoboxing:
...
It means that if we have enough memory, we could caches all the integer value(-32K-32K), which means that all the int value could be autoboxing to the same Integer object. But actually it is impractical, so we should be careful about using the following code:
Code Example
Code Block |
---|
import java.util.ArrayList; public class TestWrapper1 {  public static void main(String[] args) {   //create an array list of integers, which each element   //is more than 127     ArrayList<Integer> list1 = new ArrayList<Integer>();     for(int i=0;i<10;i++)      list1.add(i);   //create another array list of integers, which each element   //is the same with the first one     ArrayList<Integer> list2 = new ArrayList<Integer>();     for(int i=0;i<10;i++)      list2.add(i);                int counter = 0;     for(int i=0;i<10;i++)      if(list1.get(i) == list2.get(i)) counter++;     //output the total equal number     System.out.println(counter);  } } |
JDK 5.0, the output of this code is 0. But it is an undefined behavior, which depends on how many caches we could use.
Risk Assessment
The result is an undefined behavior, so it will exert a potential security risk.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
| medium | likely | low |
|
|
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Chapter 5, Core Java⢠2 Volume I - Fundamentals, Seventh Edition