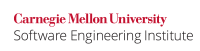
Autoboxing can automatically wrap the primitive type to the corresponding wrapper object, which can be convenient in many cases and avoid clutters in your own code. But you should always be careful about this process, especially when comparision. Considerthe follwing code:
...
Noncompliant code Example
Code Block |
---|
public class TestWrapper2 { Â public static void main(String[] args) { Â Â Â Â Integer i1 = 100; Â Â Â Â Integer i2 = 100; Â Â Â Â Integer i3 = 1000; Â Â Â Â Integer i4 = 1000; Â Â Â Â System.out.println(i1==i2); Â Â Â Â System.out.println(i3==i4); Â Â Â Â Â } } |
...
Here there exists a cache in the Integer, which clearly explains the result of above code. It also means that if we have enough memory, we could caches all the integer value(-32K-32K), which means that all the int value could be autoboxing to the same Integer object. However, actually it is impractical, so we should be careful about using the following code.
Noncompliant Code Example
In many times, you may want to create a dynamic array of integers. Unfortunately, the type parameter inside the angle brackets cannot be a primitive type. It is not possible to form an ArrayList<int>. Thanks to the wrapper class, now you can use ArrayList<Integer> to achieve this goal.
...