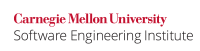
...
Inner class usage is prone to error unless the semantics are well understood. A common notion is that only the outer class can access the contents of the nested inner class(es). Not only does the inner class have access to the private fields of the outer class, the same fields can be accessed by another class in the package depending on whether the inner class is declared public or if it contains public methods/constructors.
...
Noncompliant Code Example
The code in this non-compliant noncompliant example illegally exposes the (x,y)
coordinates through the getPoint()
method of the inner class. The AnotherClass
class can thus as a result illegally access the coordinates which is clearly not desired.
Code Block | ||
---|---|---|
| ||
class Coordinates { private int x; private int y; public class Point { public void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); p.getPoint(); } } |
Compliant Solution
Use the private
access specifier for declaring the inner class(es) and all contained methods and constructors.
Code Block | ||
---|---|---|
| ||
class Coordinates { private int x; private int y; private class Point { private void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); p.getPoint(); } } |
Risk Assessment
TODO
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SCP02-J | ?? | ?? | ?? | P?? | L?? |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
JLS, 8.1.3 Inner Classes and Enclosing Instances http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.1.3
Securing Java, Gary McGraw