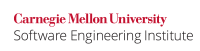
...
Code Block | ||
---|---|---|
| ||
class passwordPassword { public static void changePassword(String password_file) throws FileNotFoundException { FileInputStream fin; fin = openPasswordFile(password_file); } public static FileInputStream openPasswordFile(String password_file) throws FileNotFoundException { //Declare as final and assign before the body of the anonymous inner class //Array f[] is used to maintain language semantics while using final final FileInputStream f[]={null}; final String file = password_file; //Use own privilege to open the sensitive password file AccessController.doPrivileged(new PrivilegedAction() { public Object run() { try { f[0] = new FileInputStream("c:\\" + file); //Perform privileged action }catch(FileNotFoundException cnf) { System.err.println(cnf.getMessage()); } return null; //Still mandatory to return from run() } }); return f[0]; //Returns a reference to privileged objects (inappropriate) } } |
...
Code Block | ||
---|---|---|
| ||
class passwordPassword { public static void changePassword() { //Use own privilege to open the sensitive password file final String password_file = "password"; final FileInputStream f[] = {null}; AccessController.doPrivileged(new PrivilegedAction() { public Object run() { try { f[0] = openPasswordFile(password_file); //call the privileged method here }catch(FileNotFoundException cnf) { System.err.println(cnf.getMessage())"Error: Operation could not be performed"); } return null; } }); //Perform other operations such as password verification } public static FileInputStream openPasswordFile(String password_file) throws FileNotFoundException { FileInputStream f = new FileInputStream("c:\\" + password_file); //Perform read/write operations on password file return f; } } |
Risk Assessment
TODOInvoking doPrivileged
with too large a scope may seriously compromise the security of a Java application.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SEC01-J | ?? medium ?? | probable | ?? high | P?? | L?? |
Automated Detection
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[Gong 03|AA. Java References#Gong 03]\] |
6.4 |
Sun Secure Coding Guidelines http://java.sun.com/security/seccodeguide.html

Documentation
AccessController \[[SCG 07|AA. Java References#SCG 07]\] Guideline 6-1 Safely invoke java.security.AccessController.doPrivileged \[[API 06|AA. Java References#API 06]\] [doPrivileged method|http://java.sun.com/ |
javase/6/docs/api/java/security/AccessController.html#doPrivileged(java.security.PrivilegedAction |

)] |