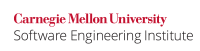
...
Code Block |
---|
class NewFinal implements Cloneable {  NewFinal(int a, int b){   this.a = a;   this.b = b;  }  void print_ab(){   System.out.println("the value a is: "+this.a);   System.out.println("the value b is: "+this.b);  }  void set_ab(int a, int b){   this.a = a;   this.b = b;  }  public NewFinal clone() throws CloneNotSupportedException{   NewFinal cloned = (NewFinal) super.clone();   return cloned;  }  private int a;  private int b; } public class Test2 {   public static void main(String[] args) {        final NewFinal mytest = new NewFinal(1,2);        mytest.print_ab();        //get the copy of original object    try {  NewFinal mytest2 = mytest.clone();        //now we change the value of a,b of the copy.   mytest2.set_ab(5, 6);   //but the original value will not be changed        mytest.print_ab();   } catch (CloneNotSupportedException e) {    // TODO Auto-generated catch block    e.printStackTrace();   }  } } |
One common mistake about this is to use public static final array, clients will be able to modify the contents of the array (although they will not be able to change the array itself, as it is final).
Non-compliant Code Example
Code Block |
---|
 public static final SomeType [] SOMETHINGS = { ... };
|
Wiki Markup |
---|
With this declaration, {{SOMETHINGS\[1\]}}, etc. can be modified by clients of the code. |
Compliant Solution
One approach is to make use of above method: first define a private array and then provide a public method that returns a copy of the array:
Code Block |
---|
private static final SomeType [] SOMETHINGS = { ... };
public static final SomeType [] somethings() {
 return SOMETHINGS.clone();
}
|
Now, the original array values cannot be modified by a client.
Compliant Solution 2
An alternative approach is to have a private array from which a public immutable list is contructed:
Code Block |
---|
private static final SomeType [] THE_THINGS = { ... };
public static final List<SomeType> SOMETHINGS =
 Collections.unmodifiableList(Arrays.asList(THE_THINGS));
|
Now, neither the original array values nor the public list can be modified by a client.
Risk Assessment
Using final to declare the reference to an object is a potential security risk, because the contents of the object can still be changed.
...