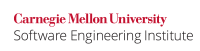
...
Synchronize on the original Collection
object when using synchronization wrappers. This synchronization is necessary to enforce atomicity (CON07-J. Do not assume that a grouping of calls to independently atomic methods is atomic).
Noncompliant Code Example (collection view)
Wiki Markup |
---|
This noncompliant code example incorrectly synchronizes on the {{set}} view of the synchronized map ({{map}}) instead of the collection object \[[Tutorials 08|AA. Java References#Tutorials 08]\]. |
Code Block | ||
---|---|---|
| ||
// map has package-private accessibility final Map<Integer, String> map = Collections.synchronizedMap(new HashMap<Integer, String>()); private final Set<Integer> set = map.keySet(); public void doSomething() { synchronized(set) { // Incorrectly synchronizes on set for (Integer k : set) { // ... } } } |
Compliant Solution (collection lock object)
This compliant solution synchronizes on the original Collection
object map
instead of the Collection
view set
.
Code Block | ||
---|---|---|
| ||
// map has package-private accessibility final Map<Integer, String> map = Collections.synchronizedMap(new HashMap<Integer, String>()); private final Set<Integer> set = map.keySet(); public void doSomething() { synchronized(map) { // Synchronize on map, not set for (Integer k : set) { // ... } } } |
Risk Assessment
Synchronizing on a collection view instead of the collection object may cause non-deterministic behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON40-J | medium | probable | medium | P8 | L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] Class Collections \[[Tutorials 08|AA. Java References#Tutorials 08]\] [Wrapper Implementations|http://java.sun.com/docs/books/tutorial/collections/implementations/wrapper.html] |
...