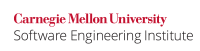
...
An alternative approach is to provide a clone
method in the class. The clone method can be used to get a copy of the original object. Now one can do anything to this new object without affecting the original object.
Code Block | ||
---|---|---|
| ||
final public class NewFinal implements Cloneable
{
NewFinal(int a, int b){
this.a = a;
this.b = b;
}
void print_ab(){
System.out.println("the value a is: "+this.a);
System.out.println("the value b is: "+this.b);
}
void set_ab(int a, int b){
this.a = a;
this.b = b;
}
public NewFinal clone() throws CloneNotSupportedException{
NewFinal cloned = (NewFinal) super.clone();
return cloned;
}
private int a;
private int b;
}
public class NewFinalCaller {
public static void main(String[] args) {
final NewFinal nf = new NewFinal(1,2);
nf.print_ab();
//get the copy of original object
try {
NewFinal nf2 = nf.clone();
//now we change the value of a,b of the copy.
nf2.set_ab(5, 6);
//but the original value will not be changed
nf.print_ab();
} catch (CloneNotSupportedException e) { e.printStackTrace(); }
}
}
|
The class is made final to prevent subclasses from overriding the clone()
method. This enables the class to be accessed and used, while preventing the fields from being modified, and complies with OBJ36-J. Provide mutable classes with a clone method.
Noncompliant Code Example
Another common mistake is to use a public static final array. Clients can trivially modify the contents of the array (although they will not be able to change the array itself, as it is final).
...
Wiki Markup |
---|
With this declaration, {{SOMETHINGS\[1\]}}, etc. can be modified by clients of the code. |
...