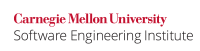
...
This code will only catch exceptions intended by the programmer to be caught. A concurrency-based exception will not be caught by this code, and can therefore be managed by code more specifically designed to handle it.
Noncompliant Code Example
In this noncompliant code example, a divide by zero exception was handled initially. Instead of the specific exception type ArithmeticException
, a more generic type Exception
was caught. This is dangerous since any future exception updates to the method signature (such as, addition of IOException
here) may no longer require the developer to provide a handler. Consequently, the recovery process may not be tailored to the specific exception type that gets thrown. Additionally, unchecked exceptions under RuntimeException
are also unintentionally caught when the top level Exception
class is caught.
Code Block | ||
---|---|---|
| ||
import java.io.IOException;
public class DivideException {
public static void main(String[] args) {
try {
division(200,5);
division(200,0); //divide by zero
} catch (Exception e) { System.out.println("Divide by zero exception : " + e.getMessage()); }
}
public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException {
int average = totalSum/totalNumber;
System.out.println("Average: "+ average);
}
}
|
Compliant Solution
To be compliant, catching specific exception types is advisable especially when the types differ significantly. Here, Arithmetic Exception
and IOException
have been unbundled as they belong to very diverse categories.
Code Block | ||
---|---|---|
| ||
import java.io.IOException;
public class DivideException {
public static void main(String[] args) {
try {
division(200,5);
division(200,0); //divide by zero
} catch (ArithmeticException ae) { throw new DivideByZeroException(); } // DivideByZeroException extends Exception so is checked
catch (IOException ie) { System.out.println("I/O Exception occurred :" + ie.getMessage()); }
}
public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException {
int average = totalSum/totalNumber;
System.out.println("Average: "+ average);
}
}
|
Exceptions
Wiki Markup |
---|
*EXC32-J-EX1*: A secure application must also abide by [EXC01-J. Do not allow exceptions to transmit sensitive information]. In order to follow this rule, an application might find it necessary to catch all exceptions at some 'top' level in order to sanitize (or suppress) them. This is also summarized in the CWE entries, [CWE 7|http://cwe.mitre.org/data/definitions/7.html] and [CWE 388|http://cwe.mitre.org/data/definitions/388.html]. If exceptions need to be caught, it is better to catch {{Throwable}} instead of {{Exception}} \[[Roubtsov 03|AA. Java References#Roubtsov 03]\]. |
...