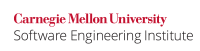
...
This noncompliant code example exposes instances of the SomeObject
class to untrusted code.
Code Block | ||
---|---|---|
| ||
public class SomeObject {
// Locks on the object's monitor
public synchronized void changeValue() {
// ...
}
}
// Untrusted code
SomeObject someObject = new SomeObject();
synchronized (someObject) {
while (true) {
// Indefinitely delay someObject
Thread.sleep(Integer.MAX_VALUE);
}
}
|
...
This noncompliant code example locks on a public nonfinal object in an attempt to use a lock other than {{SomeObject}}âs intrinsic lock.
Code Block | ||
---|---|---|
| ||
public class SomeObject {
public Object lock = new Object();
public void changeValue() {
synchronized (lock) {
// ...
}
}
}
|
...
This noncompliant code example synchronizes on a publicly accessible but nonfinal field. The lock
field is declared volatile so that changes are visible to other threads.
Code Block | ||
---|---|---|
| ||
public class SomeObject {
private volatile Object lock = new Object();
public void changeValue() {
synchronized (lock) {
// ...
}
}
public void setLock(Object lockValue) {
lock = lockValue;
}
}
|
...
This noncompliant code example uses a public final lock object.
Code Block | ||
---|---|---|
| ||
public class SomeObject {
public final Object lock = new Object();
public void changeValue() {
synchronized (lock) {
// ...
}
}
}
// Untrusted code
SomeObject someObject = new SomeObject();
someObject.lock.wait();
|
...
Thread-safe public classes that may interact with untrusted code must use a private final lock object. Existing classes that use intrinsic synchronization must be refactored to use block synchronization on such an object. In this compliant solution, calling changeValue()
obtains a lock on a private final Object
instance that is inaccessible to callers that are outside the class's scope.
Code Block | ||
---|---|---|
| ||
public class SomeObject {
private final Object lock = new Object(); // private final lock object
public void changeValue() {
synchronized (lock) { // Locks on the private Object
// ...
}
}
}
|
...
This noncompliant code example exposes the class object of SomeObject
to untrusted code.
Code Block | ||
---|---|---|
| ||
public class SomeObject {
//changeValue locks on the class object's monitor
public static synchronized void changeValue() {
// ...
}
}
// Untrusted code
synchronized (SomeObject.class) {
while (true) {
Thread.sleep(Integer.MAX_VALUE); // Indefinitely delay someObject
}
}
|
...
Thread-safe public classes that both use intrinsic synchronization over the class object and may interact with untrusted code must be refactored to use a static private final lock object and block synchronization.
Code Block | ||
---|---|---|
| ||
public class SomeObject {
private static final Object lock = new Object();
public static void changeValue() {
synchronized (lock) { // Locks on the private Object
// ...
}
}
}
|
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
LCK00-J | low | probable | medium | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Parasoft Jtest | 9.5 | TRS.SOPF | Implemented |
Related Guidelines
...
Item 52. Document Thread Safety |