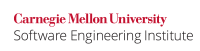
...
The ==
and !=
operators may only be used for comparing the values of boxed primitives that are not fully memoized , when the range of values represented is guaranteed to be within the ranges specified by the JLS to be fully memoized.
...
Code Block | ||
---|---|---|
| ||
public class Wrapper { public static void main(String[] args) { Integer i1 = 100; Integer i2 = 100; Integer i3 = 1000; Integer i4 = 1000; System.out.println(i1 == i2); // prints true System.out.println(i1 != i2); // prints false System.out.println(i3 == i4); // prints false System.out.println(i3 != i4); // prints true } } |
The Integer
class caches integer values from -127
to 128
only, which can result in equivalent values outside this range not comparing equal. For example, a JVM that did not cache any other values when running this program would output:
Code Block |
---|
true
false
false
true
|
Compliant Solution
This compliant solution uses the equals()
method instead of the ==
operator to compare the values of the objects. The program now prints true
, false
, true
and false
on any platform, as expected.
Code Block | ||
---|---|---|
| ||
public class Wrapper { public static void main(String[] args) { Integer i1 = 100; Integer i2 = 100; Integer i3 = 1000; Integer i4 = 1000; System.out.println(i1.equals(i2)); System.out.println(!i1.equals(i2)); System.out.println(i3.equals(i4)); System.out.println(!i3.equals(i4)); } } |
...
This noncompliant code example attempts to count the number of indices in arrays list1
and list2
that have equivalent values. Recall that class Integer
must need memoize only those integer values in the range -127 to 128; it might return non-unique objects for all values outside that range. Consequently, when comparing autoboxed integer values outside that range, the ==
operator might return false
, and the output of this example could might be 0.
Code Block | ||
---|---|---|
| ||
public class Wrapper { public static void main(String[] args) { // Create an array list of integers, where each element // is greater than 127 ArrayList<Integer> list1 = new ArrayList<Integer>(); for (int i = 0; i < 10; i++) { list1.add(i + 1000); } // Create another array list of integers, where each element // has the same value as the first list ArrayList<Integer> list2 = new ArrayList<Integer>(); for (int i = 0; i < 10; i++) { list2.add(i + 1000); } // Count matching values. int counter = 0; for (int i = 0; i < 10; i++) { if (list1.get(i) == list2.get(i)) { // uses '==' counter++; } } // print the counter: 0 in this example System.out.println(counter); } } |
...