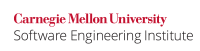
...
Code Block | ||
---|---|---|
| ||
public static void main(String[] args) throws IOException, GeneralSecurityException, ClassNotFoundException { // Build map SerializableMap<String, Integer> map = buildMap(); // Generate signing public/private key pair & sign map KeyPairGenerator kpg = KeyPairGenerator.getInstance("DSA"); KeyPair kp = kpg.generateKeyPair(); Signature sig = Signature.getInstance("SHA1withDSA"); SignedObject signedMap = new SignedObject(map, kp.getPrivate(), sig); // Generate sealing key & seal map KeyGenerator generator; generator = KeyGenerator.getInstance("AES"); generator.init(new SecureRandom()); Key key = generator.generateKey(); Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, key); SealedObject sealedMap = new SealedObject(signedMap, cipher); // Serialize map ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data")); out.writeObject(sealedMap); out.close(); // Deserialize map ObjectInputStream in = new ObjectInputStream(new FileInputStream("data")); sealedMap = (SealedObject) in.readObject(); in.close(); // Unseal map cipher = Cipher.getInstance("AES"); cipher.init(Cipher.DECRYPT_MODE, key); signedMap = (SignedObject) sealedMap.getObject(cipher); // Verify signature and retrieve map if (!signedMap.verify(kp.getPublic(), sig)) { throw new GeneralSecurityException("Map failed verification"); } map = (SerializableMap<String, Integer>) signedMap.getObject(); // Inspect map InspectMap(map); } |
Exceptions
SER02-J-EX0: A reasonable use for signing a sealed object is to certify the authenticity of a sealed object passed from elsewhere. This use represents a commitment about the sealed object itself rather than about its content [Abadi 1996].
SER02-J-EX1: Signing and sealing is required only for objects that must cross a trust boundary. Objects that never leave the trust boundary need not be signed or sealed. For example, when an entire network is contained within a trust boundary, objects that never leave that network need not be signed or sealed. Another example is objects that are only sent down a signed binary stream.
...