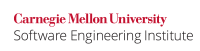
Wiki Markup |
---|
Programmers sometimes incorrectly believe that declaring a field or variable {{final}} makes the referenced object immutable. Declaring variables that have a primitive type to be {{final}} indeeddoes preventsprevent changes to their values after initialization (other than through the use of the unsupported {{sun.misc.Unsafe}} class). WhenHowever, when the variable has a reference type, however, the presence or absence of a {{final}} clause in the declaration makes _the reference itself_ immutable; the {{final}} clause lacks any effect whatsoever on the referenced object. Consequently, the fields of the referenced object can be mutable. For example, according to the Java Language Specification \[[JLS 2005|AA. Bibliography#JLS 05]\], [Section 4.12.4|http://java.sun.com/docs/books/jls/third_edition/html/typesValues.html#4.12.4], "{{final}} Variables": |
...
In this noncompliant code example, the programmer has declared the reference to the point
instance to be final
, under the incorrect assumption that he has prevented this prevents modification of the values of the instance fields x
and y
. Nevertheless, the values of the instance fields can be changed after their initialization because the final
clause applies only to the reference to the point
instance rather than to and not the referenced object.
Code Block | ||
---|---|---|
| ||
class Point { private int x; private int y; Point(int x, int y){ this.x = x; this.y = y; } void set_xy(int x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } } public class PointCaller { public static void main(String[] args) { final Point point = new Point(1, 2); point.print_xy(); // change the value of x, y point.set_xy(5, 6); point.print_xy(); } } |
When an object reference is declared final
, it signifies only that the reference cannot be changed; the mutability of the referenced contents object remains unaffected.
Compliant Solution (final
Fields)
When the values of the x
and y
members must remain immutable after their initialization, they should be declared final
. However, this requires obviates the elimination of need for the setter method set_xy()
.
...
Compliant Solution (Provide Copy Functionality)
If the class must remain mutable, another compliant solution is to provide copy functionality. This compliant solution provides a clone()
method in the final
class Point
and avoids ; avoiding the elimination of the setter method.
...
Neither the original array values nor the public
list can be modified by a client. For more details about unmodifiable wrappers, refer to guideline SEC14-J. Provide sensitive mutable classes with unmodifiable wrappers.
Risk Assessment
Using Incorrectly assuming that final
references to mutable objects can mislead unwary programmers because cause the contents of the referenced object to remain mutable can result in an attacker modifying an object thought by the programmer to be immutable.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OBJ01-J | low | probable | medium | P4 | L3 |
...