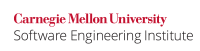
...
Code Block | ||
---|---|---|
| ||
public class Wrapper {
public static void main(String[] args) {
// Create an array list of integers, where each element
// is greater than 127
ArrayList<Integer> list1 = new ArrayList<Integer>();
for (int i = 0; i < 10; i++) {
list1.add(i + 1000);
}
// Create another array list of integers, where each element
// has the same value as the first one
ArrayList<Integer> list2 = new ArrayList<Integer>();
for (int i = 0; i < 10; i++) {
list2.add(i + 1000);
}
// Count matching values
int counter = 0;
for (int i = 0; i < 10; i++) {
if (list1.get(i).equals(list2.get(i))) { // uses 'equals()'
counter++;
}
}
// Print the counter: 10 in this example
System.out.println(counter);
}
}
|
...
Code Block | ||
---|---|---|
| ||
public void exampleEqualOperator(){ Boolean b1 = true; // Or Boolean.True Boolean b2 = true; // Or Boolean.True if (b1 == b2) { // always equal System.out.println("Will always be printedAlways print"); } } |
Exceptions
EXP03-EX0 In the unusual case where a program is guaranteed to execute only on a single implementation, it is permissible to depend on implementation-specific ranges of memoized values.
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="8bcfbe5c907f811a-6ce07864-47654a5f-a04f9f7a-09fc26633fc63b065f66832d"><ac:plain-text-body><![CDATA[ | [[Bloch 2009 | AA. Bibliography#Bloch 09]] | 4, Searching for the One | ]]></ac:plain-text-body></ac:structured-macro> | |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="938468c2962e694a-c1cdd7c5-4e8e4548-995a8e2d-f2967c315f21a774a83777b9"><ac:plain-text-body><![CDATA[ | [[JLS 2005 | AA. Bibliography#JLS 05]] | [§5.1.7, Boxing Conversion | http://java.sun.com/docs/books/jls/third_edition/html/conversions.html#5.1.7] | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="f69c297ea878295a-9f7b83b1-4c7e4e80-8c248f9e-759af57d48281497c2f9a1e6"><ac:plain-text-body><![CDATA[ | [[Pugh 2009 | AA. Bibliography#Pugh 09]] | Using == to compare objects rather than .equals | ]]></ac:plain-text-body></ac:structured-macro> |
...