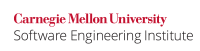
Wiki Markup |
---|
A boxing conversion converts the value of a primitive type to the corresponding value of the reference type, for instanceexample, from {{int}} to the type {{Integer}} \[[JLS 2005, Section 5.1.7|http://java.sun.com/docs/books/jls/third_edition/html/conversions.html#5.1.7], "Boxing Conversions"\]. This is convenient in many cases where an object parameter is desiredrequired, such as with collection classes like {{Map}} and {{List}}. Another use case is tofor passinteroperation objectwith referencesmethods tothat methods,require asthier opposedparameters to be object primitivereferences typesrather thatthan areprimitive alwaystypes. passedAutomatic byconversion value.to Thethe resulting wrapper types also help toreduces reduce clutter in code. |
Noncompliant Code Example
Wiki Markup |
---|
This noncompliant code example prints {{100}} as the size of the {{HashSet}} whilerather itthan isthe expected toresult print ({{1}}). The combination of values of types {{short}} and {{int}} in the operation {{i-1}} leadscauses tothe autoboxingresult ofto thebe resultautoboxed into an object of type {{Integer}}, rather than one of type {{Short}}. (See guideline [INT10-J. Be aware of integer promotion behavior].) for additional explanation of the details of the promotion rules. The {{HashSet}} contains only values of only one type {{Short}}, whereas; the code attempts to remove objects of type {{Integer}}. As a resultConsequently, the {{remove()}} operation accomplishes nothing. The compiler enforceslanguage's type checking soguarantees that only values of type {{Short}} valuescan arebe inserted into the {{HashSet}}. HoweverNevertheless, programmers aare programmerfree isto freeattempt to remove an object of _any_ type without triggering any exceptions , because {{Collections<E>.remove()}} accepts an argument of type {{Object}} rather andthan of nottype {{E}}. Such behavior can result in unintended object retention or memory leaks \[[Techtalk 2007|AA. Bibliography#Techtalk 07]\]. |
Code Block | ||
---|---|---|
| ||
public class ShortSet {
public static void main(String[] args) {
HashSet<Short> s = new HashSet<Short>();
for (short i = 0; i < 100; i++) {
s.add(i);
s.remove(i - 1);
}
System.out.println(s.size());
}
}
|
Compliant Solution
Objects removed from a collection should always share the type of the elements of the collection. Numeric promotion and autoboxing can produce unexpected object types. This compliant solution uses an explicit cast to short
that parallels the intended boxed type.
Code Block | ||
---|---|---|
| ||
public class ShortSet {
public static void main(String[] args) {
HashSet<Short> s = new HashSet<Short>();
for (short i = 0; i < 100; i++) {
s.add(i);
s.remove((short)(i - 1)); // cast to short
}
System.out.println(s.size());
}
}
|
Risk Assessment
Numeric promotion and autoboxing while removing elements from a Collection
can cause operations on the Collection
to fail silentlyAllowing autoboxing to produce objects of an unintended type can cause silent failures with some APIs, such as the Collections
library. These failures can result in unintended object retention, memory leaks, or incorrect program operation.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP11-J | low | probable | low | P6 | L2 |
Automated Detection
Detection of invocations of Collection.remove()
whose operand fails to match the type of the elements of the underlying collection is straightforward. It is possible, albeit unlikely, that some of these invocations could be intended. The remainder are heuristically likely to be in error. Automated detection for other APIs may be possible.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
Wiki Markup |
---|
\[[Core Java 2004|AA. Bibliography#Core Java 04]\] Chapter 5 \[[JLS 2005|AA. Bibliography#JLS 05]\] Section 5.1.7 \[[Techtalk 2007|AA. Bibliography#Techtalk 07]\] "The Joy of Sets" |
...