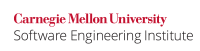
...
Code Block | ||
---|---|---|
| ||
private Boolean validateValue(long time) { // Perform validation return true; // If the time is valid } private void storeDateinDBstoreDateInDB(java.util.Date date) throws SQLException { final java.util.Date copy = (java.util.Date)date.clone(); if (validateValue(copy.getTime())) { Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... } } |
The storeDateinDBstoreDateInDB()
method accepts an untrusted date
argument and attempts to make a defensive copy using the clone()
method. The attacker can override the getTime()
method so that it returns a time that passes validation when getTime()
is called for the first time but returns an unexpected value when it is called a second time.
...
This allows an attacker to take control of the program by creating a malicious date class that extends Date
and whose clone()
method carries out the attacker's wishes. Then the attacker creates an object of this type and passes it to storeDateInDB()
.
Compliant Solution
This compliant solution avoids using the clone method. Instead, it creates a new java.util.Date
object that is subsequently used for access control checks and for insertion into the database:
Code Block | ||
---|---|---|
| ||
private void storeDateinDBstoreDateInDB(java.util.Date date) throws SQLException { final java.util.Date copy = new java.util.Date(date.getTime()); if (validateValue(copy.getTime())) { Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... } } |
...