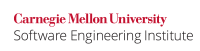
...
Code Block | ||
---|---|---|
| ||
class SuperClass {
public SuperClass () {
doLogic();
}
public void doLogic() {
System.out.println("This is superclass!");
}
}
class SubClass extends SuperClass {
private String color = null;
public SubClass() {
super();
color = "Red";
}
public void doLogic() {
// Color becomes null
System.out.println("This is subclass! The color is :" + color);
// ...
}
}
public class Overridable {
public static void main(String[] args) {
SuperClass bc = new SuperClass();
// Prints "This is superclass!"
SuperClass sc = new SubClass();
// Prints "This is subclass! The color is :null"
}
}
|
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="137d93f2d8a1974d-1c0175bf-448746d1-9ddea5df-748025a611ca0ccf98ac997b"><ac:plain-text-body><![CDATA[ | [ISO/IEC TR 24772:2010 | http://www.aitcnet.org/isai/] | Inheritance [RIP] | ]]></ac:plain-text-body></ac:structured-macro> |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="3e628761c05f22fe-11611b23-43544f6d-8538ba2e-be906e5b8200fe25bb8e2296"><ac:plain-text-body><![CDATA[ | [[ESA 2005 | AA. Bibliography#ESA 05]] | Rule 62: Do not call nonfinal methods from within a constructor | ]]></ac:plain-text-body></ac:structured-macro> | |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="af8b33f329aa89c8-a432d6f0-454644c5-b3a69799-48d3fc313418a6afb5781a4e"><ac:plain-text-body><![CDATA[ | [[JLS 2005 | AA. Bibliography#JLS 05]] | [Chapter 8, Classes | http://java.sun.com/docs/books/jls/third_edition/html/classes.html], §12.5 Creation of New Class Instances | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="bbb03d97eaf2412f-d9ca4087-46de4174-95e89782-c3cd96af097b191e88c586a3"><ac:plain-text-body><![CDATA[ | [[Rogue 2000 | AA. Bibliography#Rogue 00]] | Rule 81. Do not call non-final methods from within a constructor | ]]></ac:plain-text-body></ac:structured-macro> | |
Secure Coding Guidelines for the Java Programming Language, Version 3.0 | Guideline 4-4. Prevent constructors from calling methods that can be overridden |
...