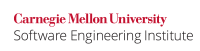
...
This noncompliant code example declares the Map
instance field volatile. The instance of the Map
object can be mutated using the is mutable, because of its put()
method; consequently, it is a mutable object.
Code Block | ||
---|---|---|
| ||
final class Foo { private volatile Map<String, String> map; public Foo() { map = new HashMap<String, String>(); // Load some useful values into map } public String get(String s) { return map.get(s); } public void put(String key, String value) { // Validate the values before inserting if (!value.matches("[\\w]*")) { throw new IllegalArgumentException(); } map.put(key, value); } } |
Interleaved calls to get()
and put()
may result in internally inconsistent values being retrieved from the Map
object because the operations within put()
modify its state. Declaring the object reference volatile is insufficient to eliminate this data race.
The put()
method lacks a time-of-check, time-of-use (TOCTOU) vulnerability, despite the presence of the validation logic, because the validation is performed on the immutable value
argument rather than on the shared Map
instance.
Noncompliant Code Example (Volatile-Read, Synchronized-Write)
...
The volatile-read, synchronized-write technique uses synchronization to preserve atomicity of compound operations, such as increment, and provides faster access times for atomic reads. However, it fails for mutable objects because the safe publication guarantee guarantee provided by volatile
extends only to the field itself (the primitive value or object reference); the referent (and hence the referent's members) is excluded from the guarantee. Consequently, the write and a subsequent read of the map
lack a happens-before relationship.
...