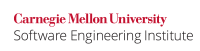
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); // wrapper int input = in.read(); if (input == -1) { throw new EOFException(); } // Down casting is permitted because InputStream guarantees read() in range // 0..255 if it is not -1 return (char)input; } public static void main(String[] args) { try { // Either redirect input from the console or use // System.setIn(new FileInputStream(""input.dat"")); System.out.print(""Enter first initial: ""); char first = getChar(); System.out.println(""Your first initial is "" + first); System.out.print(""Enter last initial: ""); char last = getChar(); System.out.println(""Your last initial is "" + last); } catch(EOFException e) { System.out.println("ERROR""ERROR"); // foward to handler } } } |
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); // This statement is now necessary to go to the next line // The noncompliant code example deceptively worked without it return (char)input; } public static void main(String[] args) { try { System.out.print(""Enter first initial: ""); char first = getChar(); System.out.println(""Your first initial is "" + first); System.out.print(""Enter last initial: ""); char last = getChar(); System.out.println(""Your last initial is "" + last); } catch(EOFException e) { System.out.println("ERROR""ERROR"); } } } |
It may appear that the mark()
and reset()
methods of BufferedInputStream
would replace the read bytes but this idea is deceptive, for, these methods provide look-ahead by operating on the internal buffers and not directly on the underlying stream.
...
FIO34-J. Do not create temporary files in shared directories 09. Input Output (FIO) FIO37-J. Do not expose buffers created using the wrap() or duplicate() methods to untrusted code