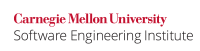
...
Code Block | ||
---|---|---|
| ||
class Key implements Serializable { // Overrides hashcode and equals methods } class HashSer { public static void main(String[] args) throws IOException, ClassNotFoundException { Hashtable<Key,String>Hashtable<Key,String> ht = new Hashtable<KeyHashtable<Key, String>String>(); Key key = new Key(); ht.put(key, "Value""Value"); System.out.println(""Entry: "" + ht.get(key)); // Retrieve using the key, works // Serialize the Hashtable object FileOutputStream fos = new FileOutputStream(""hashdata.ser""); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(ht); oos.close(); // Deserialize the Hashtable object FileInputStream fis = new FileInputStream(""hashdata.ser""); ObjectInputStream ois = new ObjectInputStream(fis); Hashtable<KeyHashtable<Key, String>String> ht_in = (Hashtable<KeyHashtable<Key, String>String>)(ois.readObject()); ois.close(); if(ht_in.contains("Value""Value")) // Check if the object actually exists in the Hashtable System.out.println(""Value was found in deserialized object.""); if (ht_in.get(key) == null) // Gets printed System.out.println(""Object was not found when retrieved using the key.""); } } |
Compliant Solution
One solution is to change the type of the key value so that it remains consistent across different runs of the program and a multitude of JVMs. This can be achieved by using an Integer
object, for example, to hold the key values.
Code Block | ||
---|---|---|
| ||
class HashSer { public static void main(String[] args) throws IOException, ClassNotFoundException { Hashtable<Integer,String>Hashtable<Integer,String> ht = new Hashtable<IntegerHashtable<Integer, String>String>(); ht.put(new Integer(1), "Value""Value"); System.out.println(""Entry: "" + ht.get(1)); // Retrieve using the key // Serialize the Hashtable object FileOutputStream fos = new FileOutputStream(""hashdata.ser""); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(ht); oos.close(); // Deserialize the Hashtable object FileInputStream fis = new FileInputStream(""hashdata.ser""); ObjectInputStream ois = new ObjectInputStream(fis); Hashtable<IntegerHashtable<Integer, String>String> ht_in = (Hashtable<IntegerHashtable<Integer, String>String>)(ois.readObject()); ois.close(); if(ht_in.contains("Value""Value")) // Check if the object actually exists in the Hashtable System.out.println(""Value was found in deserialized object.""); if (ht_in.get(1) == null) // Does not get printed System.out.println(""Object was not found when retrieved using the key.""); } } |
This problem can also be avoided by overriding the equals()
and the hashcode()
method in the Key
class, though it is best to avoid employing hash tables that are known to use implementation defined parameters.
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] Class {{Object}}, Class {{Hashtable}} \[[Bloch 08|AA. Java References#Bloch 08]\] Item 75: ""Consider using a custom serialized form"" |
...
SER35-J. Prevent overwriting of Externalizable Objects 14. Serialization (SER) SER37-J. Do not deserialize from a privileged context