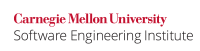
Wiki Markup |
---|
According to the Java Language Specification \[[JLS 05|AA. Java References#JLS 05]\] section 15.7 ""Evaluation Order"": |
The Java programming language guarantees that the operands of operators appear to be evaluated in a specific evaluation order, namely, from left to right.
...
Code Block | ||
---|---|---|
| ||
class BadPrecedence { public static void main(String[] args) { int number = 17; int[] threshold = new int[20]; threshold[0] = 10; number = (number >> threshold[0]? 0 : -2) + ((31 * ++number) * (number = get())); // ... if(number == 0) { System.out.println(""Access granted""); } else { System.out.println(""Denied access""); // number = -2 } } public static int get() { int number = 0; // Assign number to non zero value if authorized else 0 return number; } } |
...
Code Block | ||
---|---|---|
| ||
number = ((31 * ++number) * (number=get())) + (number >> threshold[0]? 0 : -2); |
Although this solution solves the problem, in general it is advisable to avoid using expressions with more than one side-effect. It is also inadvisable to depend on the left-right ordering for evaluation of side-effects because operands are evaluated in place first, and then subject to laws of operator precedence and associativity.
...
Code Block | ||
---|---|---|
| ||
number = ((31 * (number + 1)) * get()) + (get() >> threshold[0]? 0 : -2); |
Risk Assessment
...
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] Section 15.7 ""Evaluation Order"" and 15.7.3 ""Evaluation Respects Parentheses and Precedence"" |
...
EXP09EXP10-J. Use parentheses for precedence of operation Understand the evaluation of expressions containing non short-circuit operators 04. Expressions (EXP) EXP31-J. Avoid side effects in assertions