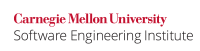
...
Code Block | ||
---|---|---|
| ||
class Password { private static void changePassword() { // Use own privilege to open the sensitive password file final String password_file = "password""password"; final FileInputStream f[] = {null}; AccessController.doPrivileged(new PrivilegedAction() { public Object run() { try { f[0] = openPasswordFile(password_file); // call the privileged method here } catch(FileNotFoundException cnf) { // cannot recover if password file is not found; log to file } return null; } }); //Perform other operations such as old password verification } private static FileInputStream openPasswordFile(String password_file) throws FileNotFoundException { FileInputStream f = new FileInputStream(password_file); // Perform read/write operations on password file return f; } } |
...
Code Block | ||
---|---|---|
| ||
public static void readFont() throws FileNotFoundException { // Use own privilege to open the font file final String font_file = "fontfile""fontfile"; try { final InputStream in = AccessController.doPrivileged(new PrivilegedExceptionAction<InputStream>PrivilegedExceptionAction<InputStream>() { public InputStream run() throws FileNotFoundException { return openFontFile(font_file); //call the privileged method here } }); // Perform other operations } catch (PrivilegedActionException exc) { Exception cause = exc.getException(); if (cause instanceof FileNotFoundException) { throw (FileNotFoundException)cause; } else { throw new Error(""Unexpected exception type"", cause); } } } |
In summary, if the code can throw a checked exception without leaking sensitive information, prefer the form of doPrivileged
method that takes a PrivilegedExceptionAction
instead of a PrivilegedAction
.
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] [method doPrivileged()|http://java.sun.com/javase/6/docs/api/java/security/AccessController.html#doPrivileged(java.security.PrivilegedAction)] \[[Gong 03|AA. Java References#Gong 03]\] Sections 6.4, AccessController and 9.5 Privileged Code \[[SCG 07|AA. Java References#SCG 07]\] Guideline 6-1 Safely invoke java.security.AccessController.doPrivileged \[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 266|http://cwe.mitre.org/data/definitions/266.html] ""Incorrect Privilege Assignment"", [CWE ID 272|http://cwe.mitre.org/data/definitions/272.html] ""Least Privilege Violation"" |
...
SEC30-J. Define wrappers around native methods 02. Platform Security (SEC) SEC32-J. Create and sign a SignedObject before creating a SealedObject