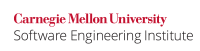
Returning references to internal mutable members of a class can seriously compromise the security of an application because of the resulting sub par encapsulation properties and susceptibility to corruption of the class data. A caller is able to can modify the private
data if instead of defensive copies of mutable class members, direct references to them are returned.
...
Code Block | ||
---|---|---|
| ||
class ReturnRef { // Internal state, may contain sensitive data Hashtable<Integer,String>Hashtable<Integer,String> ht = new Hashtable<Integer,String>Hashtable<Integer,String>(); private ReturnRef() { ht.put(1, ""123-45-6666""); } public Hashtable<Integer,String>Hashtable<Integer,String> getValues(){ return ht; } public static void main(String[] args) { ReturnRef rr = new ReturnRef(); Hashtable<IntegerHashtable<Integer, String>String> ht1 = rr.getValues(); // Prints sensitive data 123-45-6666 ht1.remove(1); // Untrusted caller can remove entries Hashtable<IntegerHashtable<Integer, String>String> ht2 = rr.getValues(); // Now prints null, original entry is removed } } |
...
Code Block | ||
---|---|---|
| ||
private Hashtable<Integer,String>Hashtable<Integer,String> getValues(){ return (Hashtable<Integer,String>Hashtable<Integer,String>)ht.clone(); // shallow copy } public static void main(String[] args) { ReturnRef rr = new ReturnRef(); Hashtable<Integer,String>Hashtable<Integer,String> ht1 = rr.getValues(); // prints non sensitive data ht1.remove(1); // untrusted caller can remove entries only from the copy Hashtable<Integer,String>Hashtable<Integer,String> ht2 = rr.getValues(); // prints non sensitive data } |
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as OBJ35-CPP. Do not return references to private data.
...
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] [method clone()|http://java.sun.com/javase/6/docs/api/java/lang/Object.html#clone()] \[[Security 06|AA. Java References#Security 06]\] \[[Bloch 08|AA. Java References#Bloch 08]\] Item 39: Make defensive copies when needed \[[SCG 07|AA. Java References#SCG 07]\] Guideline 2-1 Create a copy of mutable inputs and outputs \[[Haggar 00|AA. Java References#Haggar 00]\] [Practical Java Praxis 64: Use clone for Immutable Objects When Passing or Receiving Object References to Mutable Objects|http://www.informit.com/articles/article.aspx?p=20530] \[[Goetz 06|AA. Java References#Goetz 06]\] 3.2. Publication and Escape: Allowing Internal Mutable State to Escape \[[Gong 03|AA. Java References#Gong 03]\] 9.4 Private Object State and Object Immutability \[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 375|http://cwe.mitre.org/data/definitions/375.html] ""Passing Mutable Objects to an Untrusted Method"" |
...
OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely 08. Object Orientation (OBJ) OBJ38-J. Immutable classes must prohibit extension