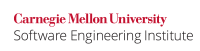
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); // wrapper int input = in.read(); if (input == -1) { throw new EOFException(); } // Down casting is permitted because InputStream guarantees read() in range // 0..255 if it is not -1 return (char)input; } public static void main(String[] args) { try { // Either redirect input from the console or use // System.setIn(new FileInputStream(""input.dat"")); System.out.print(""Enter first initial: ""); char first = getChar(); System.out.println(""Your first initial is "" + first); System.out.print(""Enter last initial: ""); char last = getChar(); System.out.println(""Your last initial is "" + last); } catch(EOFException e) { System.out.println("ERROR""ERROR"); // foward to handler } } } |
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); // This statement is now necessary to go to the next line // The noncompliant code example deceptively worked without it return (char)input; } public static void main(String[] args) { try { System.out.print(""Enter first initial: ""); char first = getChar(); System.out.println(""Your first initial is "" + first); System.out.print(""Enter last initial: ""); char last = getChar(); System.out.println(""Your last initial is "" + last); } catch(EOFException e) { System.out.println("ERROR""ERROR"); } } } |
It may appear that the mark()
and reset()
methods of BufferedInputStream
would replace the read bytes but this idea is deceptive, for, these methods provide look-ahead by operating on the internal buffers and not directly on the underlying stream.
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] [method read|http://java.sun.com/javase/6/docs/api/java/io/InputStream.html#read()] \[[API 06|AA. Java References#API 06]\] [class BufferedInputStream|http://java.sun.com/javase/6/docs/api/java/io/BufferedInputStream.html] |
...
IDS00-J. Always validate user input 09. Input Output (FIO) 11. Concurrency (CON)