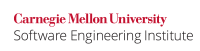
...
Wiki Markup |
---|
According to the Java Language Specification \[[JLS 05|AA. Java References#JLS 05]\], section 4.12.4 ""{{final}} Variables"": |
... if a
final
variable holds a reference to an array, then the components of the array may be changed by operations on the array, but the variable will always refer to the same array.
...
Code Block | ||
---|---|---|
| ||
class FinalClass{ private int a; private int b; FinalClass(int a, int b){ this.a = a; this.b = b; } void set_ab(int a, int b){ this.a = a; this.b = b; } void print_ab(){ System.out.println(""the value a is: "" + this.a); System.out.println(""the value b is: "" + this.b); } } public class FinalCaller { public static void main(String[] args) { final FinalClass fc = new FinalClass(1, 2); fc.print_ab(); // change the value of a,b. fc.set_ab(5, 6); fc.print_ab(); } } |
...
Code Block | ||
---|---|---|
| ||
final public class NewFinal implements Cloneable { private int a; private int b; NewFinal(int a, int b){ this.a = a; this.b = b; } void print_ab(){ System.out.println(""the value a is: ""+ this.a); System.out.println(""the value b is: ""+ this.b); } void set_ab(int a, int b){ this.a = a; this.b = b; } public NewFinal clone() throws CloneNotSupportedException{ NewFinal cloned = (NewFinal) super.clone(); return cloned; } } public class NewFinalCaller { public static void main(String[] args) throws CloneNotSupportedException { final NewFinal nf = new NewFinal(1, 2); nf.print_ab(); // Get the copy of original object NewFinal nf2 = nf.clone(); // Change the value of a,b of the copy. nf2.set_ab(5, 6); // Original value will not be changed nf.print_ab(); } } |
...
Code Block | ||
---|---|---|
| ||
private static final String[] items = { ... }; public static final List<String>List<String> itemsList = Collections.unmodifiableList(Arrays.asList(items)); |
...
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] Sections 4.12.4 ""final Variables"" and 6.6, ""Access Control"" \[[Bloch 08|AA. Java References#Bloch 08]\] Item 13: Minimize the accessibility of classes and members \[[Core Java 04|AA. Java References#Core Java 04]\] Chapter 6 \[[MITRE 09|AA. Java References#MITRE 09]\] [CWE ID 607|http://cwe.mitre.org/data/definitions/607.html] ""Public Static Final Field References Mutable Object"" |
...
OBJ02-J. Avoid using finalizers 08. Object Orientation (OBJ) OBJ04-J. Encapsulate the absence of an object by using a Null Object