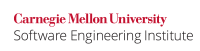
...
This noncompliant code example uses a thread-safe class Book
that cannot be modified by a client.
Code Block |
---|
final class Book { // May change its locking policy in the future to use private internal locks private final String title; private Calendar dateIssued; private Calendar dateDue; Book(String title) { this.title = title; } public synchronized void issue(int days) { dateIssued = Calendar.getInstance(); dateDue = Calendar.getInstance(); dateDue.add(Calendar.DAY_OF_MONTH, days); } public synchronized Calendar getDueDate() { return dateDue; } } |
Furthermore, the This class does not commit to its locking strategy and . That is, it reserves the right to change its locking strategy without notice. Furthermore, it also does not document that callers can safely use client-side locking. The client class BookWrapper
uses client-side locking in the renewBookrenew()
method by synchronizing on a Book
instance.
...
If class Book
changes its synchronization policy in the future, the BookWrapper
class's locking strategy will silently breakmight silently break. For instance, the Bookwrapper
locking strategy will definitely break if Book
is modified to use an internal private lock, as recommended by CON04-J. Synchronize using an internal private lock object. This is because threads that call getDueDate()
of class BookWrapper
may perform operations on the thread-safe Book
using its new locking policy (internal private lock), however, threads that call method renew()
will always synchronize on the intrinsic lock of the Book
instance. Consequently, the implementation will use two different locks.
...