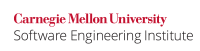
It is highly unlikely that a method is built to deal with all possible runtime exceptions. Consequently, no method should ever catch RuntimeException
. If a method catches RuntimeException
, it may receive exceptions it was not designed to handle, such as NullPointerException
. Many catch
clauses simply log or ignore their errorthe enclosed exceptional condition, and normal execution resumes. Runtime exceptions indicate bugs in the program that should be fixed by the developer. They almost always lead to control flow vulnerabilities. Likewise, a method should never catch Exception
or Throwable
, because this implies catching RuntimeException
.
Noncompliant Code Example
The following function This noncompliant code example takes a String
argument and returns true
if it consists of a capital letter succeeded by lowercase letters. To handle corner cases, it merely wraps the code in a try-catch
block and reports any exceptions that may arise.
Code Block | ||
---|---|---|
| ||
boolean isCapitalized(String s) {
try {
if (s.equals("")) {
return true;
}
String first = s.substring( 0, 1);
String rest = s.substring( 1);
return (first.equals (first.toUpperCase()) &&
rest.equals (rest.toLowerCase()));
} catch (RuntimeException exception) {
ExceptionReporter.report (exception);
}
return false;
}
|
This code reports errors if s
is a null pointer. However, it also unintentionally catches other errors exceptional conditions that are unlikely to be handled properly, such as if the string belongs to a different is being modified concurrently by another thread.
Compliant Solution
...