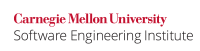
...
This code reports errors if s
is a null pointer. However, it also unintentionally catches other exceptional conditions that are unlikely to be handled properly, such as if the string is being modified concurrently by another threadan index is out of bounds.
Compliant Solution
Instead of catching RuntimeException
, a program should be as specific in catching exceptions as possible.
...
This code only catches those exceptions that are intended to be caught. For example, a concurrency based an index-out-of-bounds exception is not caught.
Noncompliant Code Example
In this This noncompliant code example , handles a divide by zero exception is handled initially. Instead of the specific exception type ArithmeticException
, a more generic type Exception
is caught. This is dangerous as any future exception declaration updates to the method signature (such as, addition of IOException
) may no longer require the developer to provide a handler. Consequently, the recovery process may not be tailored to the specific exception type that is thrown. Additionally, unchecked exceptions under RuntimeException
are also unintentionally caught whenever the top level Exception
class is caught.
...
Noncompliant Code Example
This noncompliant code example improves by catching a specific divide-by-zero exception but fails on the premise that it unscrupulously accepts other undesirable runtime exceptions, by catching Exception
.
Code Block | ||
---|---|---|
| ||
try { division(200,5); division(200,0); //divide Divide by zero } catch (ArithmeticException ae) { throw new DivideByZeroException(); } // DivideByZeroException extends Exception so is checked catch (Exception e) { System.out.println("Exception occurred :" + e.getMessage()); } |
Compliant Solution
To be compliant, catching catch specific exception types is advisable especially when the types differ significantly. Here, Arithmetic Exception
ArithmeticException
and IOException
have been unbundled as because they belong to fall under very diverse categories.
Code Block | ||
---|---|---|
| ||
import java.io.IOException; public class DivideException { public static void main(String[] args) { try { division(200,5); division(200,0); //divide Divide by zero } catch (ArithmeticException ae) { throw new DivideByZeroException(); } // DivideByZeroException extends Exception so is checked catch (IOException ie) { System.out.println("I/O Exception occurred :" + ie.getMessage()); } } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average = totalSum/totalNumber; // Additional operations that may throw IOException... System.out.println("Average: "+ average); } } |
...