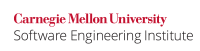
...
Prefer using the block form of synchronization when there are nonatomic operations within the method that do not require any synchronization. These operations can be decoupled from those that require synchronization and executed outside the synchronized block. You should also consider using an internal private lock object, as recommended by CON04-J. Synchronize using an internal private lock object.
Noncompliant Code Example (synchronizedList)
This noncompliant code example comprises an ArrayList
collection which is non-thread-safe by default. However, most thread-unsafe classes have a synchronized thread-safe version, for example, Collections.synchronizedList
is a good substitute for ArrayList
and Collections.synchronizedMap
is a good alternative to HashMap
. The atomicity pitfall described in the coming linessubsequent paragraph, remains to be addressed even when the particular Collection
offers thread-safety benefits.
...
Code Block | ||
---|---|---|
| ||
public class KeyedCounter { private final Map<String,Integer> map = new HashMap<String,Integer>(); private final Object lock = new Object(); public void increment(String key) { synchronized (lock) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue() + 1; map.put(key, value); } } public Integer getCount(String key) { synchronized (lock) { return map.get(key); } } } |
Also, note that this would be a violation of a previously discussed noncompliant code example if the field map
were to refer to a Collections.synchronizedMap
object. This compliant solution uses the intrinsic lock of the class for all purposesNote that this solution does not use Collections.synchronizedMap()
. This is because locking on the (unsynchronized) map provides sufficient thread-safety for this application. The rule CON02-J. Always synchronize on the appropriate object indicates certain objects that are not to be synchronized on, and this includes any object returned by Collections.synchronizedMap()
.
Compliant Solution (ConcurrentHashMap
)
...