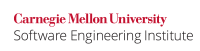
...
This guideline applies to all uses of Collection
classes including the thread-safe Hashtable
class. Enumerations of the objects of a Collection
and iterators also require explicit synchronization on the Collection
object or any single lock object.
Noncompliant Code Example (synchronizedList)
This noncompliant code example comprises an ArrayList
collection which is non-thread-safe by default. There is, however, a way around this drawback. Most thread-unsafe classes have a synchronized thread-safe version, for example, Collections.synchronizedList
is a good substitute for ArrayList
and Collections.synchronizedMap
is a good alternative to HashMap
. The atomicity pitfall described in the coming lines, remains to be addressed even when the particular Collection
offers thread-safety benefits.
...
When the addAndPrintIP()
method is invoked on the same object from multiple threads, the output, consisting of varying array lengths, indicates a race condition between the threads. In other words, the statements that are responsible for adding an IP address and printing it out are not sequentially consistent. Also note that the operations within a thread's run()
method are non-atomic.
Noncompliant Code Example (Subclass)
This noncompliant code example extends the base class and synchronizes on the addAndPrintIP()
method which is required to be atomic.
...
Wiki Markup |
---|
Moreover, when a wrapper such as {{Collections.synchronizedList()}} is used (as shown in the previous noncompliant code example), it is unwieldy for a client to determine the type of the class ({{List}}) that is being wrapped. Consequently, it is not directly possible to extend the class \[[Goetz 06|AA. Java References#Goetz 06]\]. |
Noncompliant Code Example (Method synchronization)
This noncompliant code example appears to use synchronization when defining the addAndPrintIP()
method, however, it acquires an intrinsic lock instead of the lock of the List
object. This means that another thread may modify the value of the List
instance when the addAndPrintIP()
method is executing.
...
This noncompliant code example also violates OBJ00-J. Declare data members private.
Compliant Solution (Synchronized block)
Wiki Markup |
---|
To eliminate the race condition, ensure atomicity by using the underlying list's lock. This can be achieved by including all statements that use the array list within a synchronized block that locks on the list. This technique is also called client-side locking \[[Goetz 06|AA. Java References#Goetz 06]\]. |
...
Wiki Markup |
---|
Although expensive, {{CopyOnWriteArrayList}} and {{CopyOnWriteArraySet}} classes are sometimes used to create copies of the core {{Collection}} so that iterators do not fail with a runtime exception when some data in the {{Collection}} is modified. However, any updates to the {{Collection}} are not immediately visible to other threads. Consequently, their use is limited to boosting performance in code where the writes are fewer (or non-existent) as compared to the reads \[[JavaThreads 04|AA. Java References#JavaThreads 04]\]. In all other cases they must be avoided. |
Compliant Solution (Composition)
Composition offers more benefits as compared to the previous solution, although at the cost of a slight performance penalty (refer to OBJ07-J. Understand how a superclass can affect a subclass for details on how to implement composition).
...
Wiki Markup |
---|
This approach allows the {{CompositeCollection}} class to use its own intrinsic lock in a way that is completely independent of the lock of the underlying list class. Moreover, this permits the underlying collection to be thread-unsafe because the {{CompositeCollection}} wrapper prevents direct accesses to its methods by exposing its own synchronized equivalents. This approach also provides consistent locking even when the underlying list is not thread-safe or when it changes its locking policy. \[[Goetz 06|AA. Java References#Goetz 06]\] |
Noncompliant Code Example (synchronizedMap)
Wiki Markup |
---|
This noncompliant code example defines a thread-unsafe {{KeyedCounter}} class. Even though the {{HashMap}} field is synchronized, the overall {{increment}} operation is not atomic. \[[Lee 09|AA. Java References#Lee 09]\] |
Code Block | ||
---|---|---|
| ||
public class KeyedCounter { private Map<String, Integer> map = Collections.synchronizedMap(new HashMap<String, Integer>()); public void increment(String key) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue() + 1; map.put(key, value); } public Integer getCount(String key) { return map.get(key); } } |
Compliant Solution (Synchronized method)
Wiki Markup |
---|
This compliant solution declares the {{increment()}} method as {{synchronized}} to ensure atomicity \[[Lee 09|AA. Java References#Lee 09]\]. |
...
Also, note that this would be a violation of a previously discussed noncompliant code example if the field map
were to refer to a Collections.synchronizedMap
object. This compliant solution uses the intrinsic lock of the class for all purposes.
Compliant Solution (ConcurrentHashMap)
Wiki Markup |
---|
The previous compliant solution does not scale very well because a class with several {{synchronized}} methods is a potential bottleneck as far as acquiring locks is concerned and may further lead to contention or deadlock. The class {{ConcurrentHashMap}}, through a more preferable approach, provides several utility methods to perform atomic operations and is used in this compliant solution \[[Lee 09|AA. Java References#Lee 09]\]. According to Goetz et al. \[[Goetz 06|AA. Java References#Goetz 06]\] section 5.2.1. ConcurrentHashMap: |
...
Code Block | ||
---|---|---|
| ||
public class KeyedCounter { private final ConcurrentMap<String, AtomicInteger> map = new ConcurrentHashMap<String, AtomicInteger>(); public void increment(String key) { AtomicInteger value = new AtomicInteger(0); AtomicInteger old = map.putIfAbsent(key, value); if (old != null) { value = old; } value.incrementAndGet(); // Increment the value atomically } public Integer getCount(String key) { AtomicInteger value = map.get(key); return (value == null) ? null : value.get(); } } |
Risk Assessment
Non-atomic code can induce race conditions and affect program correctness.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON07- J | low | probable | medium | P4 | L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] Class Vector, Class WeakReference \[[JavaThreads 04|AA. Java References#JavaThreads 04]\] 8.2 "Synchronization and Collection Classes" \[[Goetz 06|AA. Java References#Goetz 06]\] 4.4.1. Client-side Locking, 4.4.2. Composition and 5.2.1. ConcurrentHashMap \[[Lee 09|AA. Java References#Lee 09]\] "Map & Compound Operation" |
...