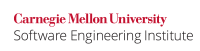
...
Code Block | ||
---|---|---|
| ||
class BankAccount implements Comparable<BankAccount> { private int balanceAmount; // Total amount in bank account private final Object lock; private final long id; // unique for each BankAccount private static long NextIDnextID = 0; // next unused id private BankAccount(int balance) { this.balanceAmount = balance; this.lock = new Object(); this.id = this.NextIDnextID++; } public int compareTo(BankAccount ba) { if (this.id < ba.id) { return -1; } if (this.id > ba.id) { return 1; } return 0; } // Deposits the amount from this object instance to BankAccount instance argument ba private void depositAllAmount(BankAccount ba) { BankAccount former, latter; if (compareTo(ba) < 0) { former = this; latter = ba; } else { former = ba; latter = this; } synchronized (former) { synchronized (latter) { ba.balanceAmount += this.balanceAmount; this.balanceAmount = 0; // withdraw all amount from this instance ba.displayAllAmount(); // Display the new balanceAmount in ba (may cause deadlock) } } } private synchronized void displayAllAmount() { System.out.println(balanceAmount); } public static void initiateTransfer(final BankAccount first, final BankAccount second) { Thread t = new Thread(new Runnable() { public void run() { first.depositAllAmount(second); } }); t.start(); } } |
...