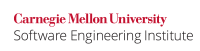
...
This noncompliant code example involves the method sendPage()
that sends a Page
object containing information being passed between a client and a server. The method is synchronized to protect access to the array pageBuff
. Calling writeObject()
within the synchronized sendPage
can result in a deadlock for condition in high latency networks or lossy when network connections are inherently lossy.
Code Block | ||
---|---|---|
| ||
// Class Page is defined separately. It stores and returns the Page name via getName()
public final boolean SUCCESS = true;
public final boolean FAILURE = false;
Page[] pageBuff = new Page[MAX_PAGE_SIZE];
public synchronized boolean sendPage(Socket socket, String pageName) throws IOException {
// Get the output stream to write the Page to
ObjectOutputStream out = new ObjectOutputStream(socket.getOutputStream());
Page targetPage = null;
// Find the Page requested by the client (this operation requires synchronization)
for(Page p : pageBuff) {
if(p.getName().compareTo(pageName) == 0) {
targetPage = p;
}
}
// Page requested does not exist
if(targetPage == null) {
return FAILURE;
}
// Send the Page to the client (does not require any synchronization)
out.writeObject(targetPage);
out.flush();
out.close();
return SUCCESS;
}
|
...
- Perform actions on data structures requiring synchronization
- Create copies of the objects that are required to sendbe sent
- Perform network calls in a separate method that does not require any synchronization
In this compliant solution, the synchronized method getPage()
is called from SendReplysendReply()
to find the appropriate Page
requested by the client from the array pageBuff
of type Page
. The method sendReply()
in turn calls the unsynchronized method sendPage()
to deliver the Page
.
...