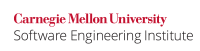
As the java.io.InputStream
class is abstract
, a wrapper such as BufferedInputStream
is required to provide a concrete implementation that overrides its methods. Java input classes, for example Scanner
and BufferedInputStream
, often buffer the underlying input stream to facilitate fast, non-blocking I/O. As the InputStream
class is abstract
, a wrapper such as BufferedInputStream
is required to provide a concrete implementation that overrides its methods. It is permissible to create multiple wrappers on an InputStream
. Programs that encourage multiple wrappers around the same stream, however, behave significantly different depending on whether the InputStream
allows look-ahead or not. An adversary can exploit this difference in behavior by, for example, redirecting System.in
(from a file). This is also possible when a program uses the System.setIn()
method to redirect System.in
. That said, redirecting input from the console is a standard practice in UNIX based platforms but finds limited application in others such as Windows, where console programs are largely considered outmoded. In general, any input stream that supports non-blocking buffered I/O is susceptible to misuse.
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); // wrapper int input = in.read(); if (input == -1) { throw new EOFException(); } // downDown casting is permitted because InputStream guarantees read() in range // 0..255 if it is not -1 return (char)input; } public static void main(String[] args) { try { // Either redirect input from the console or use // System.setIn(new FileInputStream("input.dat")); System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); // foward to handler } } } |
Implementation Details
This program was compiled with the command javac InputLibrary.java
on a system with Java 1.6.0. When run from the command line with java InputLibrary
, the program successfully takes two characters as input and prints them out. However, when run with java InputLibrary < input
, where input
is a file that contains the exact same input, the program prints "ERROR" throws an EOFException
because the second call to getChar()
finds no characters to read upon encountering the end of the stream.
...
Code Block | ||
---|---|---|
| ||
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); // This statement is now necessary to go to the next line // The Noncompliantnoncompliant code example deceptively worked without it return (char)input; } public static void main(String[] args) { try { System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); } } } |
...